mirror of
https://github.com/meshtastic/meshtastic.git
synced 2025-03-05 21:00:08 -08:00
updated hardware devices
This commit is contained in:
parent
438c1cd5d9
commit
313dcc6341
|
@ -5,6 +5,176 @@ sidebar_label: Setup GPIO Peripherals
|
|||
sidebar_position: 6
|
||||
---
|
||||
|
||||
import Peripherals from '../hardware/peripheral/index.mdx'
|
||||
|
||||
<Peripherals components={props.components} />
|
||||
## Firmware Versions
|
||||
|
||||
:::warning
|
||||
GPIO access is fundamentally dangerous because invalid options can physically damage or destroy your hardware. Ensure that you fully understand the schematic for your particular device before trying this as we do not offer a warranty. Use at your own risk.
|
||||
:::
|
||||
|
||||
The device firmware runs on the nodes to build the mesh for communication. Each different make and model of device requires a different build of the Meshtastic firmware in order to run properly. Thankfully, due to the design of Meshtastic, it is possible to port the firmware to new devices as they become available. The firmware currently runs on a range of ESP32 based devices, but there is also increasing support for the nRF52 microprocessor with some more recent devices coming to market.
|
||||
|
||||
The current firmware has support for a screen to display received messages, along with information about nodes on the mesh, and more detailed information about the device on which it is running.
|
||||
|
||||
The latest firmware can be downloaded from the [Downloads](/downloads) page. If you wish to view the code or contribute to development of the firmware, please visit the device code [GitHub page](https://github.com/meshtastic/firmware).
|
||||
|
||||
:::info
|
||||
Please be aware that there are significant changes between version branches 1.2.x and 1.3.x which mean that devices need to be running the same branch of firmware to be able to talk to each other. Python, Android, and other software applications will also need to be running the same branch to be able to talk to the device.
|
||||
|
||||
This feature uses a preinstalled module in the device code and associated command line flags/classes in the python code. You'll need to be running at least version 1.2.23 (or later) of the python and device code to use this feature.
|
||||
:::
|
||||
|
||||
## Remote Hardware
|
||||
|
||||
### Supported Operations
|
||||
|
||||
- Set any GPIO
|
||||
- Read any GPIO
|
||||
- Receive notification of changes in any GPIO
|
||||
|
||||
### Setup
|
||||
|
||||
You can get the latest python tool/library with `pip3 install --upgrade meshtastic` on Windows/Linux/OS-X. See the [python section](/docs/software/python/cli/installation) for more details.
|
||||
|
||||
To prevent access from untrusted users, you must first make a `gpio` channel that is used for authenticated access to this feature. You'll need to install this channel on both the local and remote node.
|
||||
|
||||
The procedure using the python command line tool is:
|
||||
|
||||
1. Connect local device via USB
|
||||
2. Create a GPIO channel:
|
||||
```shell
|
||||
meshtastic --ch-add gpio
|
||||
```
|
||||
3. If doing local testing, you may also want to change the speed of the channel:
|
||||
```sh
|
||||
meshtastic --ch-mediumfast
|
||||
```
|
||||
4. Check the channel has been created and copy the long "Complete URL" that contains all the channels on that device:
|
||||
```shell
|
||||
meshtastic --info
|
||||
```
|
||||
5. Connect the remote device via USB (or use the [remote admin](/docs/configuration/remote-admin) feature to reach it through the mesh)
|
||||
6. Set it to join the gpio channel you created:
|
||||
```shell
|
||||
meshtastic --seturl theurlyoucopiedinstep3
|
||||
```
|
||||
|
||||
Now both devices should be able to talk over the `gpio` channel. Send a text message from one the other other verify. Also run `--nodes` to verify the second node shows up.
|
||||
|
||||
### Masks
|
||||
|
||||
To determine the appropriate mask for the pin(s) that you want to know. The python program (and output) below might help:
|
||||
|
||||
```python
|
||||
>>> for i in range(1,45):
|
||||
... print(f'GPIO:{i} mask:{hex(2**i)}')
|
||||
...
|
||||
GPIO:1 mask:0x2
|
||||
GPIO:2 mask:0x4
|
||||
GPIO:3 mask:0x8
|
||||
GPIO:4 mask:0x10
|
||||
GPIO:5 mask:0x20
|
||||
GPIO:6 mask:0x40
|
||||
GPIO:7 mask:0x80
|
||||
GPIO:8 mask:0x100
|
||||
GPIO:9 mask:0x200
|
||||
GPIO:10 mask:0x400
|
||||
GPIO:11 mask:0x800
|
||||
GPIO:12 mask:0x1000
|
||||
GPIO:13 mask:0x2000
|
||||
GPIO:14 mask:0x4000
|
||||
GPIO:15 mask:0x8000
|
||||
GPIO:16 mask:0x10000
|
||||
GPIO:17 mask:0x20000
|
||||
GPIO:18 mask:0x40000
|
||||
GPIO:19 mask:0x80000
|
||||
GPIO:20 mask:0x100000
|
||||
GPIO:21 mask:0x200000
|
||||
GPIO:22 mask:0x400000
|
||||
GPIO:23 mask:0x800000
|
||||
GPIO:24 mask:0x1000000
|
||||
GPIO:25 mask:0x2000000
|
||||
GPIO:26 mask:0x4000000
|
||||
GPIO:27 mask:0x8000000
|
||||
GPIO:28 mask:0x10000000
|
||||
GPIO:29 mask:0x20000000
|
||||
GPIO:30 mask:0x40000000
|
||||
GPIO:31 mask:0x80000000
|
||||
GPIO:32 mask:0x100000000
|
||||
GPIO:33 mask:0x200000000
|
||||
GPIO:34 mask:0x400000000
|
||||
GPIO:35 mask:0x800000000
|
||||
GPIO:36 mask:0x1000000000
|
||||
GPIO:37 mask:0x2000000000
|
||||
GPIO:38 mask:0x4000000000
|
||||
GPIO:39 mask:0x8000000000
|
||||
GPIO:40 mask:0x10000000000
|
||||
GPIO:41 mask:0x20000000000
|
||||
GPIO:42 mask:0x40000000000
|
||||
GPIO:43 mask:0x80000000000
|
||||
GPIO:44 mask:0x100000000000
|
||||
```
|
||||
|
||||
## Testing GPIO Operations
|
||||
|
||||
You can programmatically do operations from your own python code by using the Meshtastic `RemoteHardwareClient` class. See the [Python API](/docs/software/python/cli/installation) documentation for more details.
|
||||
|
||||
You can add a simple LED and resistor to validate that the GPIO operations work as expected. Use [this tutorial](https://www.instructables.com/Slide-Switch-With-Arduino-Uno-R3/) as a guide.
|
||||
|
||||
### Requirements
|
||||
|
||||
- (x2) Meshtastic devices (one device could be on a local computer, and the other one just has to be powered and is the one with the LED to be connected to it)
|
||||
- (x2) wires (black and yellow; they can be any color but typically black is used for ground)
|
||||
- (x1) LED
|
||||
- (x1) 220Ω resistor (somewhat optional, but recommended)
|
||||
- (x1) Breadboard (optional)
|
||||
|
||||
### Preparation
|
||||
|
||||
1. Disconnect the remote device from power (battery/usb)
|
||||
2. Add a resistor from yellow wire to the one end of the LED (either end of the resistor is OK, either end of the LED is OK)
|
||||
3. Add the yellow wire from a GPIO pin that will not cause any issues (ex: for TLoraV1, we can use GPIO21)
|
||||
4. Add the black "ground" wire from the ground pin on the device (ex: for TLoraV1 it is the end pin next to the RST button) to the other end of the LED
|
||||
5. Power on the device
|
||||
|
||||
### Validation
|
||||
|
||||
By default, the pin may be "off" or "on". (It will most likely "off".) See the steps below for running commands. In the example of GPIO21, the mask would be `0x200000`.
|
||||
|
||||

|
||||
|
||||
|
||||
## Using GPIOs from the Python CLI
|
||||
|
||||
### Writing a GPIO
|
||||
|
||||
```shell title="Example: turning 'on' GPIO4"
|
||||
meshtastic --port /dev/ttyUSB0 --gpio-wrb 4 1 --dest 28979058
|
||||
# Connected to radio
|
||||
# Writing GPIO mask 0x10 with value 0x10 to !28979058
|
||||
```
|
||||
|
||||
### Reading a GPIO
|
||||
|
||||
```shell title="Example: read GPIO4"
|
||||
meshtastic --port /dev/ttyUSB0 --gpio-rd 0x10 --dest 28979058
|
||||
# Connected to radio
|
||||
# Reading GPIO mask 0x10 from !28979058
|
||||
# GPIO read response gpio_value=16
|
||||
```
|
||||
|
||||
:::note
|
||||
If the mask and the gpio_value match, then the value is "on". If the gpio_value is 0, then the value is "off".
|
||||
:::
|
||||
|
||||
### Watching for GPIO Changes
|
||||
|
||||
```shell title="Example: watching GPIO4 for changes"
|
||||
meshtastic --port /dev/ttyUSB0 --gpio-watch 0x10 --dest 28979058
|
||||
# Connected to radio
|
||||
# Watching GPIO mask 0x10 from !28979058
|
||||
# Received RemoteHardware typ=GPIOS_CHANGED, gpio_value=16
|
||||
# Received RemoteHardware typ=GPIOS_CHANGED, gpio_value=0
|
||||
# Received RemoteHardware typ=GPIOS_CHANGED, gpio_value=16
|
||||
# < press ctrl-c to exit >
|
||||
```
|
|
@ -1,6 +1,6 @@
|
|||
label: Antennas
|
||||
collapsible: true
|
||||
position: 1
|
||||
position: 2
|
||||
link:
|
||||
type: generated-index
|
||||
title: Antennas
|
|
@ -1,116 +0,0 @@
|
|||
---
|
||||
id: lora
|
||||
title: LILYGO® TTGO Lora devices
|
||||
sidebar_label: LILYGO® Lora
|
||||
sidebar_position: 6
|
||||
---
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
LILYGO® Lora23 v1-2.1 * Versions other than 2.1 not recommended with a battery
|
||||
|
||||
<Tabs
|
||||
groupId="t-lora"
|
||||
defaultValue="v1"
|
||||
values={[
|
||||
{label: 'Lora V1', value: 'v1'},
|
||||
{label: 'Lora V1.3', value: 'v1.3'},
|
||||
{label: 'Lora V2.0', value: 'v2.0'},
|
||||
{label: 'Lora V2.1-1.6', value:'v2.1'}
|
||||
]}>
|
||||
<TabItem value="v1">
|
||||
|
||||
- ESP32 - WiFi & Bluetooth
|
||||
- SX1276 - LoRa Transceiver
|
||||
- Frequency options:
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- Built in 0.96 inch OLED display
|
||||
- U.FL antenna connector
|
||||
- Reset and Program switches
|
||||
- No GPS
|
||||
|
||||
- Firmware file: `firmware-tlora-v1-1.x.x.bin`
|
||||
- [Purchase link](https://www.aliexpress.com/item/32840238513.html)
|
||||
|
||||
[<img alt="LILYGO® TTGO Lora V1" src="/img/hardware/lora-v1.png" style={{zoom:'25%'}} />](/img/hardware/lora-v1.png)
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="v1.3">
|
||||
|
||||
- ESP32 - WiFi & Bluetooth
|
||||
- SX127x - LoRa Transceiver
|
||||
- Frequency options:
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- Built in 0.96 inch OLED display
|
||||
- U.FL antenna connector
|
||||
- Reset and Program switches
|
||||
- No GPS
|
||||
|
||||
|
||||
- Firmware file: `firmware-tlora_v1_3-1.x.x.bin`
|
||||
- [Purchase link](https://www.aliexpress.com/item/4000628100802.html)
|
||||
|
||||
|
||||
[<img alt="LILYGO® TTGO Lora V1.3" src="/img/hardware/lora-v1.3.png" style={{zoom:'25%'}} />](/img/hardware/lora-v1.3.png)
|
||||
[<img alt="LILYGO® TTGO Lora V1.3 pin map" src="/img/hardware/lora-v1.3_pinmap.webp" style={{zoom:'25%'}} />](/img/hardware/lora-v1.3_pinmap.webp)
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="v2.0">
|
||||
|
||||
- ESP32 - WiFi & Bluetooth
|
||||
- SX127x - LoRa Transceiver
|
||||
- Frequency options:
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- Built in 0.96 inch OLED display
|
||||
- U.FL antenna connector
|
||||
- Power and Reset switches
|
||||
- microSD connector
|
||||
- No GPS
|
||||
|
||||
|
||||
- Firmware file: `firmware-tlora-v2-1.x.x.bin`
|
||||
- [Purchase link](https://www.aliexpress.com/item/32846302183.html)
|
||||
|
||||
|
||||
[<img alt="LILYGO® TTGO Lora V2" src="/img/hardware/lora-v2.0.png" style={{zoom:'25%'}} />](/img/hardware/lora-v2.0.png)
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="v2.1">
|
||||
|
||||
- ESP32 - WiFi & Bluetooth
|
||||
- SX127x - LoRa Transceiver
|
||||
- Frequency options:
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- Built in 0.96 inch OLED display
|
||||
- SMA antenna connector
|
||||
- Power and Reset switches
|
||||
- microSD connector
|
||||
- No GPS
|
||||
|
||||
|
||||
- Firmware file: `firmware-tlora-v2-1-1.6-1.x.x.bin`
|
||||
- [Purchase link](https://www.aliexpress.com/item/32915894264.html)
|
||||
|
||||
<br />
|
||||
|
||||
:::warning
|
||||
Early versions of some of these boards contained the wrong component in the LiPo battery charging circuit allowing the battery to be overcharged.
|
||||
:::
|
||||
|
||||
[<img src="/img/hardware/lora-v2.1-1.6.png" alt="LILYGO® TTGO Lora V2.1-1.6" style={{zoom:'25%'}} />](/img/hardware/lora-v2.1-1.6.png)
|
||||
|
||||
Shorting IO12 to ground will progress the screen pages, wake up the device, etc. A simple push switch can be added for this purpose.
|
||||
|
||||
[<img src="/img/hardware/lora32-v2-1.6-button.jpg" style={{zoom:'30%'}} />](/img/hardware/lora32-v2-1.6-button.jpg)
|
||||
[<img src="/img/hardware/lora32-v2-1.6-button-example.jpg" style={{zoom:'30%'}} />](/img/hardware/lora32-v2-1.6-button.jpg)
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
||||
|
||||
Further information on the LILYGO® LoRa and T-beam devices can be found on LILYGO®'s [GitHub page](https://github.com/Xinyuan-LilyGO/LilyGo-LoRa-Series).
|
25
docs/hardware/devices/lora/buttons.mdx
Normal file
25
docs/hardware/devices/lora/buttons.mdx
Normal file
|
@ -0,0 +1,25 @@
|
|||
---
|
||||
id: buttons
|
||||
title: Buttons
|
||||
sidebar_label: Buttons
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
## Functionality
|
||||
|
||||
- **Reset Button**
|
||||
- _**Single press**_ resets the device.
|
||||
- _**Double press**_ sets the Device in bootloader mode and mounts a drive to your computer (nRF52 devices only)
|
||||
- **Program button**
|
||||
- _**Single press**_ changes the page of information displayed on the screen.
|
||||
- _**Double press**_ sets the Bluetooth pairing code to `123456`.
|
||||
- _**Long press**_ adjusts the contrast of the screen.
|
||||
- _**Long press during reboot**_ turns on the software WiFi access point.
|
||||
|
||||
|
||||
## GPIO IO12 - TTGO Lora V2.1-1.6
|
||||
|
||||
Shorting IO12 to ground will progress the screen pages, wake up the device, etc. A simple push switch can be added for this purpose.
|
||||
|
||||
[<img src="/img/hardware/lora32-v2-1.6-button.jpg" style={{zoom:'30%'}} />](/img/hardware/lora32-v2-1.6-button.jpg)
|
||||
[<img src="/img/hardware/lora32-v2-1.6-button-example.jpg" style={{zoom:'30%'}} />](/img/hardware/lora32-v2-1.6-button.jpg)
|
26
docs/hardware/devices/lora/enclosures.mdx
Normal file
26
docs/hardware/devices/lora/enclosures.mdx
Normal file
|
@ -0,0 +1,26 @@
|
|||
---
|
||||
id: enclosures
|
||||
title: Enclosures
|
||||
sidebar_label: Enclosures
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
import Tropho from '/img/enclosures/3dp-tropho-lora32.png';
|
||||
|
||||
## 3D Printed
|
||||
|
||||
### Created by tropho/TonyG
|
||||
|
||||
#### TTGO LoRa32 v2.1.1.6 Case
|
||||
|
||||
Download from [Printables](https://www.printables.com/model/131389-ttgo-lora32-v2116-case-for-meshtastic) or purchase from the creator's [Etsy Store](https://www.etsy.com/listing/1285837219/ttgo-lora-21-16-case).
|
||||
|
||||
##### Required Hardware
|
||||
|
||||
- (x4) M3x16mm socket-head cap screws
|
||||
- (x4) M3 Nuts
|
||||
- (x2) M2 Screws (to secure TTGO LoRa32 board to frame)
|
||||
- (x1) LiPo battery pack (1,000mAh)
|
||||
- (x1) Momentary micro push button for PRG (6x6x5mm)
|
||||
|
||||
<img src={Tropho} width="600" align="left" />
|
144
docs/hardware/devices/lora/index.mdx
Normal file
144
docs/hardware/devices/lora/index.mdx
Normal file
|
@ -0,0 +1,144 @@
|
|||
---
|
||||
id: lora
|
||||
title: LILYGO® TTGO Lora Devices
|
||||
sidebar_label: LILYGO® Lora
|
||||
sidebar_position: 6
|
||||
---
|
||||
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
Further information on the LILYGO® LoRa devices can be found on LILYGO®'s [GitHub page](https://github.com/Xinyuan-LilyGO/LilyGo-LoRa-Series).
|
||||
|
||||
<Tabs
|
||||
groupId="t-lora"
|
||||
defaultValue="v1"
|
||||
values={[
|
||||
{label: 'Lora V1', value: 'v1'},
|
||||
{label: 'Lora V1.3', value: 'v1.3'},
|
||||
{label: 'Lora V2.0', value: 'v2.0'},
|
||||
{label: 'Lora V2.1-1.6', value:'v2.1'}
|
||||
]}>
|
||||
<TabItem value="v1">
|
||||
|
||||
:::warning
|
||||
Not recommended with a battery! These boards contain the wrong component in the LiPo battery charging circuit allowing the battery to be overcharged.
|
||||
:::
|
||||
|
||||
- **MCU**
|
||||
- ESP32 (WiFi & Bluetooth)
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX1276
|
||||
- **Frequency options**
|
||||
- 915 MHz
|
||||
- 868 MHz
|
||||
- **Connectors**
|
||||
- Micro USB
|
||||
- Antenna: U.FL antenna connector
|
||||
|
||||
**Features**
|
||||
- Built in 0.96 inch OLED display
|
||||
|
||||
**Resources**
|
||||
- Firmware file: `firmware-tlora-v1-X.X.X.xxxxxxx.bin`
|
||||
- Purchase link: [AliExpress](https://www.aliexpress.com/item/32840238513.html)
|
||||
|
||||
|
||||

|
||||
|
||||
</TabItem>
|
||||
<TabItem value="v1.3">
|
||||
|
||||
:::warning
|
||||
Not recommended with a battery! These boards contain the wrong component in the LiPo battery charging circuit allowing the battery to be overcharged.
|
||||
:::
|
||||
|
||||
- **MCU**
|
||||
- ESP32 (WiFi & Bluetooth)
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX127x
|
||||
- **Frequency options**
|
||||
- 915 MHz
|
||||
- 868 MHz
|
||||
- **Connectors**
|
||||
- Micro USB
|
||||
- Antenna: U.FL antenna connector
|
||||
|
||||
**Features**
|
||||
- Built in 0.96 inch OLED display
|
||||
|
||||
**Resources**
|
||||
- Firmware file: `firmware-tlora_v1_3-X.X.X.xxxxxxx.bin`
|
||||
- Purchase link: [AliExpress](https://www.aliexpress.com/item/4000628100802.html)
|
||||
|
||||

|
||||

|
||||
|
||||
</TabItem>
|
||||
<TabItem value="v2.0">
|
||||
|
||||
|
||||
:::warning
|
||||
Not recommended with a battery! These boards contain the wrong component in the LiPo battery charging circuit allowing the battery to be overcharged.
|
||||
:::
|
||||
|
||||
|
||||
- **MCU**
|
||||
- ESP32 (WiFi & Bluetooth)
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX127x
|
||||
- **Frequency options**
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- **Connectors**
|
||||
- Micro USB
|
||||
- Antenna: U.FL antenna connector
|
||||
|
||||
**Features**
|
||||
- Built in 0.96 inch OLED display
|
||||
- Power and Reset switches
|
||||
- microSD connector
|
||||
- No GPS
|
||||
|
||||
**Resources**
|
||||
- Firmware file: `firmware-tlora-v2-X.X.X.xxxxxxx.bin`
|
||||
- Purchase link: [AliExpress](https://www.aliexpress.com/item/32846302183.html)
|
||||
|
||||

|
||||
|
||||
</TabItem>
|
||||
<TabItem value="v2.1">
|
||||
|
||||
|
||||
:::caution
|
||||
Not recommended with a battery. Early versions of some of these boards contained the wrong component in the LiPo battery charging circuit allowing the battery to be overcharged.
|
||||
:::
|
||||
|
||||
- **MCU**
|
||||
- ESP32 (WiFi & Bluetooth)
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX127x
|
||||
- **Frequency options**
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- **Connectors**
|
||||
- Micro USB
|
||||
- Antenna: SMA antenna connector
|
||||
|
||||
**Features**
|
||||
- Built in 0.96 inch OLED display
|
||||
- Power and Reset switches
|
||||
- microSD connector
|
||||
- No GPS
|
||||
|
||||
**Resources**
|
||||
- Firmware file: `firmware-tlora-v2-1-1.6-X.X.X.xxxxxxx.bin`
|
||||
- Purchase link: [AliExpress](https://www.aliexpress.com/item/32915894264.html)
|
||||
|
||||
|
||||

|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
|
@ -1,33 +0,0 @@
|
|||
---
|
||||
id: nano-g1
|
||||
title: Nano G1 device
|
||||
sidebar_label: Nano G1
|
||||
sidebar_position: 4
|
||||
---
|
||||
|
||||
The Nano G1 is the first dedicated hardware to be designed from scratch purely for Meshtastic by Neil Hao. It has been designed to be small and compact with the inclusion of a high quality internal PCB antenna.
|
||||
|
||||
Only the US 915 MHz version is available currently. There should be an EU 868 MHz version available in the future.
|
||||
|
||||
### Features
|
||||
|
||||
- Meshtastic pre-installed
|
||||
- ESP32 WROOM microprocessor - WiFi & Bluetooth
|
||||
- Semtech SX1276 - LoRa Transceiver
|
||||
- Frequency options:
|
||||
- 915 MHz
|
||||
- Additional ultra-low noise amplifier to improve LoRa receiver sensitivity
|
||||
- ATGM336H-5N-71 Whole Constellation Positioning and Navigation Module (Supports GPS, BDS and GLONASS)
|
||||
- Built in 915Mhz Lora PCB Antenna (VSWR <=1.5 @ 915 MHz)
|
||||
- User button
|
||||
- 1.3 inch OLED screen
|
||||
- Buzzer
|
||||
|
||||
### Resources
|
||||
|
||||
- Firmware file: `firmware-nano-g1-1.x.x.bin`
|
||||
- [Purchase link](https://www.tindie.com/products/neilhao/meshtastic-mesh-device-nano-edition/)
|
||||
|
||||
[<img alt="Nano G1" src="/img/hardware/nano-g1-front.jpg" style={{zoom:'25%'}} />](/img/hardware/nano-g1-front.jpg)
|
||||
|
||||
Further information on the Nano G1 can be found on [Unit Engineering's Wiki](https://uniteng.com/wiki/doku.php?id=meshtastic:nano).
|
14
docs/hardware/devices/nano-g1/buttons.mdx
Normal file
14
docs/hardware/devices/nano-g1/buttons.mdx
Normal file
|
@ -0,0 +1,14 @@
|
|||
---
|
||||
id: buttons
|
||||
title: Buttons
|
||||
sidebar_label: Buttons
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
## Functionality
|
||||
|
||||
- **Program Button**
|
||||
- _**Single press**_ changes the page of information displayed on the screen.
|
||||
- _**Double press**_ sets the Bluetooth pairing code to 123456.
|
||||
- _**Long press**_ adjusts the contrast of the screen.
|
||||
- _**Long press during reboot**_ turns on the software WiFi access point.
|
41
docs/hardware/devices/nano-g1/index.mdx
Normal file
41
docs/hardware/devices/nano-g1/index.mdx
Normal file
|
@ -0,0 +1,41 @@
|
|||
---
|
||||
id: nano-g1
|
||||
title: Nano G1 device
|
||||
sidebar_label: Nano G1
|
||||
sidebar_position: 4
|
||||
---
|
||||
|
||||
The Nano G1 is the first dedicated hardware device to be designed from scratch purely for Meshtastic by Neil Hao. It has been designed to be small and compact with the inclusion of a high quality internal PCB antenna.
|
||||
|
||||
### Specifications
|
||||
|
||||
- **MCU**
|
||||
- ESP32 WROOM (WiFi & Bluetooth)
|
||||
- Bluetooth 4.2
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX1276
|
||||
- Additional ultra-low noise amplifier to improve LoRa receiver sensitivity
|
||||
- **Frequency options**
|
||||
- US-915 MHz
|
||||
- **Navigation Module**
|
||||
- ATGM336H-5N-71 (Supports GPS, BDS and GLONASS)
|
||||
- **Antenna**
|
||||
- Built in 915Mhz Lora PCB Antenna (VSWR <=1.5 @ 915 MHz)
|
||||
- **Connectors**
|
||||
- USB-C
|
||||
|
||||
### Features
|
||||
|
||||
- Meshtastic pre-installed
|
||||
- User button
|
||||
- 1.3 inch OLED screen
|
||||
- Buzzer
|
||||
|
||||
### Resources
|
||||
|
||||
- Firmware file: `firmware-nano-g1-1.x.x.bin`
|
||||
- [Purchase link](https://www.tindie.com/products/neilhao/meshtastic-mesh-device-nano-edition/)
|
||||
|
||||

|
||||
|
||||
Further information on the Nano G1 can be found on [Unit Engineering's Wiki](https://uniteng.com/wiki/doku.php?id=meshtastic:nano).
|
140
docs/hardware/devices/rak/base-boards.mdx
Normal file
140
docs/hardware/devices/rak/base-boards.mdx
Normal file
|
@ -0,0 +1,140 @@
|
|||
---
|
||||
id: base-board
|
||||
title: RAK WisBlock Base Boards
|
||||
sidebar_label: Base Boards
|
||||
sidebar_position: 1
|
||||
---
|
||||
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
## WisBlock Base
|
||||
|
||||
<Tabs
|
||||
groupId="rakbase board"
|
||||
defaultValue="RAK19007"
|
||||
values={[
|
||||
{label: 'RAK5005-O', value: 'RAK5005-O'},
|
||||
{label: 'RAK19007', value:'RAK19007'},
|
||||
{label: 'RAK19003', value: 'RAK19003'},
|
||||
{label: 'RAK19001', value: 'RAK19001'}
|
||||
]}>
|
||||
<TabItem value="RAK5005-O">
|
||||
|
||||
### RAK5005-O
|
||||
|
||||
- **Board**
|
||||
- [RAK5005-O](https://store.rakwireless.com/products/rak5005-o-base-board) - The original WisBlock Base Board.
|
||||
- **Slots**
|
||||
- (x1) Core Module slot
|
||||
- (x1) WisBlock IO Module slot
|
||||
- (x4) WisBlock Sensor Module slots
|
||||
- **Buttons**
|
||||
- (x1) Reset Button
|
||||
- It may be possible to add a user button using the [13002 IO module](https://store.rakwireless.com/collections/wisblock-interface/products/adapter-module-rak13002).
|
||||
- **Connectors**
|
||||
- Connector for 3.7v LiPo battery (with charge controller)
|
||||
- Connector for 5v solar panel (max 5.5v)
|
||||
- I<sup>2</sup>C, UART, GPIOs and analog input accessible with solder contacts
|
||||
- Micro USB port for debugging and power
|
||||
- **Screen Support**
|
||||
- OLED screen support (OLED screen sold separately)
|
||||
|
||||
|
||||
Further information on the RAK5005-O can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK5005-O/Overview/#product-description).
|
||||
|
||||
<img
|
||||
alt="RAK4631 5005"
|
||||
src="/img/hardware/rak4631_5005.png"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
</TabItem>
|
||||
|
||||
<TabItem value="RAK19007">
|
||||
|
||||
### RAK19007
|
||||
|
||||
- **Board**
|
||||
- [RAK19007](https://store.rakwireless.com/products/rak19007-wisblock-base-board-2nd-gen) - WisBlock Base Board (2nd Generation, an upgrade to the RAK5005-O)
|
||||
- **Slots**
|
||||
- (x1) Core Module slot
|
||||
- (x1) WisBlock IO Module slot
|
||||
- (x4) WisBlock Sensor Module slots
|
||||
- **Buttons**
|
||||
- (x1) Reset Button
|
||||
- It may be possible to add a user button using the [13002 IO module](https://store.rakwireless.com/collections/wisblock-interface/products/adapter-module-rak13002).
|
||||
- **Connectors**
|
||||
- Connector for 3.7v LiPo battery (with charge controller)
|
||||
- Connector for 5v solar panel (max 5.5v)
|
||||
- I<sup>2</sup>C, UART, BOOT and GPIOs accessible with solder contacts
|
||||
- USB-C port for debugging and power
|
||||
- **Screen Support**
|
||||
- OLED screen support (OLED screen sold separately)
|
||||
|
||||
|
||||
Further information on the RAK19007 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK19007/Overview/#product-description).
|
||||
|
||||
|
||||
</TabItem>
|
||||
|
||||
<TabItem value="RAK19003">
|
||||
|
||||
### RAK19003
|
||||
|
||||
- **Board**
|
||||
- [RAK19003](https://store.rakwireless.com/products/wisblock-base-board-rak19003) - WisBlock's Mini Base Board.
|
||||
- **Slots**
|
||||
- (x1) Core Module slot
|
||||
- (x1) WisBlock IO Module slot
|
||||
- (x2) WisBlock Sensor Module slots
|
||||
- **Buttons**
|
||||
- (x1) Reset Button
|
||||
- It is currently not possible to add a user button to this board.
|
||||
- **Connectors**
|
||||
- Connector for 3.7v LiPo battery (with charge controller)
|
||||
- Connector for 5v solar panel (max 5.5v)
|
||||
- I<sup>2</sup>C, UART and BOOT headers accessible with solder contacts
|
||||
- Micro USB port for debugging and power
|
||||
- **Screen Support**
|
||||
- OLED screen support (OLED screen sold separately)
|
||||
|
||||
|
||||
Further information on the RAK19003 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK19003/Overview/#product-description)
|
||||
|
||||
<img
|
||||
alt="RAK4631 19003"
|
||||
src="/img/hardware/rak4631_19003.png"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="RAK19001">
|
||||
|
||||
### RAK19001
|
||||
|
||||
- **Board**
|
||||
- [RAK19001](https://store.rakwireless.com/products/rak19001-wisblock-dual-io-base-board) - WisBlock's Dual IO Base Board.
|
||||
- **Slots**
|
||||
- (x1) Core Module slot
|
||||
- (x2) WisBlock IO Module slot
|
||||
- (x6) WisBlock Sensor Module slots
|
||||
- **Buttons**
|
||||
- (x1) Reset Button
|
||||
- (x1) User-defined push button switch
|
||||
- (x1) Battery selector switch
|
||||
- It may be possible to add a user button using the [13002 IO module](https://store.rakwireless.com/collections/wisblock-interface/products/adapter-module-rak13002).
|
||||
- **Connectors**
|
||||
- Connector for 3.7v LiPo battery (with charge controller)
|
||||
- Separate connector for non-rechargeable batteries
|
||||
- Connector for 5v solar panel (max 5.5v)
|
||||
- I<sup>2</sup>C, SPI, UART, BOOT and GPIOs accessible with solder contacts
|
||||
- USB-C port for debugging and power
|
||||
- **Screen Support**
|
||||
- OLED screen support (OLED screen sold separately)
|
||||
|
||||
|
||||
Further information on the RAK19001 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK19001/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
21
docs/hardware/devices/rak/buttons.mdx
Normal file
21
docs/hardware/devices/rak/buttons.mdx
Normal file
|
@ -0,0 +1,21 @@
|
|||
---
|
||||
id: buttons
|
||||
title: Buttons
|
||||
sidebar_label: Buttons
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
## Functionality
|
||||
|
||||
Button functionality for RAK devices greatly depends on the device specific configuration. If your device has any of the following buttons, the functionality is generally the same for all RAK devices:
|
||||
|
||||
- **Reset Button**
|
||||
- _**Single press**_ resets the device.
|
||||
- _**Double press**_ sets the Device in bootloader mode and mounts a drive to your computer (nRF52 devices only)
|
||||
- **Power Button**
|
||||
- _**Long press**_ powers the device off or turns it back on again.
|
||||
- **Program button**
|
||||
- _**Single press**_ changes the page of information displayed on the screen.
|
||||
- _**Double press**_ sets the Bluetooth pairing code to `123456` (useful if you do not have a screen on the device).
|
||||
- _**Long press**_ adjusts the contrast of the screen.
|
||||
- _**Long press during reboot**_ turns on the software WiFi access point on devices that support WiFi. (ESP32 devices only)
|
102
docs/hardware/devices/rak/core-modules.mdx
Normal file
102
docs/hardware/devices/rak/core-modules.mdx
Normal file
|
@ -0,0 +1,102 @@
|
|||
---
|
||||
id: core-module
|
||||
title: RAK WisBlock Core Modules
|
||||
sidebar_label: Core Modules
|
||||
sidebar_position: 2
|
||||
---
|
||||
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
## WisBlock Core
|
||||
|
||||
<Tabs
|
||||
groupId="rakcore"
|
||||
defaultValue="RAK4631"
|
||||
values={[
|
||||
{label: 'RAK4631', value: 'RAK4631'},
|
||||
{label: 'RAK11200', value: 'RAK11200'}
|
||||
]}>
|
||||
<TabItem value="RAK4631">
|
||||
|
||||
### RAK4631
|
||||
|
||||
- **MCU** nRF52840
|
||||
- Bluetooth BLE 5.0
|
||||
- Very low power consumption
|
||||
- **Meshtastic Firmware**
|
||||
- [`firmware-rak4631-2.X.X.xxxxxxx.uf2`](/downloads)
|
||||
- **LoRa transceiver**
|
||||
- SX1262
|
||||
- **Frequency Options**
|
||||
- 433 MHz
|
||||
- 470 MHz
|
||||
- 799 MHz
|
||||
- 865 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 920 MHz
|
||||
- 923 MHz
|
||||
- **Connectors**
|
||||
- U.FL antenna
|
||||
|
||||
|
||||
Please be aware of the difference between the RAK4631 and the RAK4631-R which uses the RUI3 bootloader which is incompatible with Meshtastic. The RAK4631 uses the Arduino bootloader that is required for Meshtastic. If you have a RAK4631-R, please see the [instructions for converting the bootloader](/docs/guides/convert-rak4631r).
|
||||
|
||||
Further information on the RAK4631 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK4631/Overview/#product-description).
|
||||
|
||||
<img
|
||||
alt="RAK4631 Core Module"
|
||||
src="/img/hardware/rak4631.png"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="RAK11200">
|
||||
|
||||
|
||||
### RAK11200 / RAK13300
|
||||
|
||||
:::caution Note
|
||||
Only supported on the RAK5005-O base board.
|
||||
:::
|
||||
|
||||
The RAK11200 does not contain a LoRa transceiver, and thus needs to be added separately in the form of the [RAK13300 LPWAN module](https://store.rakwireless.com/products/rak13300-wisblock-lpwan). This occupies the IO Port of the base board.
|
||||
|
||||
- **RAK11200**
|
||||
- **MCU** ESP32-WROVER
|
||||
- Bluetooth 4.2
|
||||
- WiFi 802.11 b/g/n
|
||||
- High power consumption
|
||||
- **Meshtastic Firmware**
|
||||
- [`firmware-rak11200-2.X.X.xxxxxx.bin`](/downloads)
|
||||
|
||||
|
||||
Further information on the RAK11200 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK11200/Overview/#product-description).
|
||||
|
||||
- **RAK13300**
|
||||
- **LoRa transceiver**
|
||||
- SX1262
|
||||
- **Frequency Options**
|
||||
- 433 MHz
|
||||
- 470 MHz
|
||||
- 864 MHz
|
||||
- 865 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 920 MHz
|
||||
- 923 MHz
|
||||
- **Connectors**
|
||||
- U.FL antenna
|
||||
|
||||
|
||||
Further information on the RAK13300 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK13300/Overview/#product-description).
|
||||
|
||||
<img
|
||||
alt="RAK4631 5005 11200"
|
||||
src="/img/hardware/rak11200.jpg"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
32
docs/hardware/devices/rak/enclosures.mdx
Normal file
32
docs/hardware/devices/rak/enclosures.mdx
Normal file
|
@ -0,0 +1,32 @@
|
|||
---
|
||||
id: enclosures
|
||||
title: Enclosures
|
||||
sidebar_label: Enclosures
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
## Created by tropho/TonyG
|
||||
|
||||
### RAK5005 Case
|
||||
|
||||
Download from [Printables](https://www.printables.com/model/286651-rak5005-case-for-meshtastic) or purchase from the creator's [Etsy Store](https://www.etsy.com/listing/1158237722/meshtastic-rak5005-case).
|
||||
|
||||

|
||||
|
||||
### RAK19007 Case
|
||||
|
||||
Download from [Printables](https://www.printables.com/model/286657-rak19007-case-for-meshtastic) or purchase from the creator's [Etsy Store](https://www.etsy.com/listing/1302265084/meshtastic-rak19007-case).
|
||||
|
||||

|
||||
|
||||
### RAK19003 Case
|
||||
|
||||
Download from [Printables](https://www.printables.com/model/286662-rak19003-case-for-meshtastic) or purchase from the creator's [Etsy Store](https://www.etsy.com/listing/1316287559/meshtastic-rak19003-case).
|
||||
|
||||

|
||||
|
||||
### RAK19003 (Micro) Case
|
||||
|
||||
Download from [Printables](https://www.printables.com/model/286664-rak19003-micro-case-for-meshtastic) or purchase from the creator's [Etsy Store](https://www.etsy.com/listing/1302260756/meshtastic-rak19003-micro-case).
|
||||
|
||||

|
26
docs/hardware/devices/rak/index.mdx
Normal file
26
docs/hardware/devices/rak/index.mdx
Normal file
|
@ -0,0 +1,26 @@
|
|||
---
|
||||
id: wisBlock
|
||||
title: RAK WisBlock Devices
|
||||
sidebar_label: RAK WisBlock
|
||||
sidebar_position: 1
|
||||
---
|
||||
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
<!-- RAK LOGO here? -->
|
||||
|
||||
The RAK WisBlock is a modular hardware system that can be used to build Meshtastic devices.
|
||||
|
||||
RAK Wireless currently sells a [Meshtastic Starter kit](https://store.rakwireless.com/products/wisblock-meshtastic-starter-kit) that has the minimum you need to get started.
|
||||
|
||||
If you wish to purchase parts separately, you will need a [WisBlock Base Board](/docs/hardware/devices/rak/base) and a [WisBlock Core Module](/docs/hardware/devices/rak/core). Please ensure you choose the correct operating frequency for your country when purchasing.
|
||||
|
||||
You can optionally purchase peripherals such as a GPS module, Screen, Sensor, or other various modules.
|
||||
|
||||
Please see the RAK documentation for the correct way to connect your hardware to ensure that you do not damage the device. There is currently no pin required to pair RAK devices via BLE.
|
||||
|
||||
## Resources
|
||||
|
||||
- RAK's Wisblock [Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock)
|
||||
- RAK's [GitHub Page](https://github.com/RAKWireless/WisBlock) for the WisBlock
|
76
docs/hardware/devices/rak/peripherals.mdx
Normal file
76
docs/hardware/devices/rak/peripherals.mdx
Normal file
|
@ -0,0 +1,76 @@
|
|||
---
|
||||
id: peripherals
|
||||
title: Supported Peripherals
|
||||
sidebar_label: Peripherals
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
<Tabs
|
||||
groupId="rakmodules"
|
||||
defaultValue="GPS"
|
||||
values={[
|
||||
{label: 'GPS Module', value: 'GPS'},
|
||||
{label: 'Buzzer', value: 'Buzzer'},
|
||||
{label: 'I/O Module', value: 'IO'},
|
||||
{label: 'Environmental Sensor', value:'BME680'}
|
||||
]}>
|
||||
<TabItem value="GPS">
|
||||
|
||||
To add a GPS to the RAK5005-O base board, you need the [RAK1910 GPS sensor](https://store.rakwireless.com/collections/wisblock-sensor/products/rak1910-max-7q-gnss-location-sensor). It is supported on slot A of the 5005 board via UART.
|
||||
|
||||
- uBlox MAX-7Q GPS module
|
||||
- GPS and GLONASS satellite support
|
||||
|
||||
|
||||
Further information on the RAK1910 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK1910/Overview/#product-description).
|
||||
|
||||
To add a GPS to the RAK19003 base board, you need the [RAK12500 GPS sensor](https://store.rakwireless.com/products/wisblock-gnss-location-module-rak12500). It is supported via I<sup>2</sup>C on slot B for firmware versions 1.49 and above.
|
||||
|
||||
- uBlox Zoe-M8Q GNSS receiver
|
||||
- GPS, GLONASS, QZSS and BeiDou satellite support
|
||||
|
||||
|
||||
Further information on the RAK12500 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK12500/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="Buzzer">
|
||||
|
||||
The [RAK18001 Buzzer Module](https://store.rakwireless.com/products/wisblock-buzzer-module-rak18001) is currently being tested for integration with the External Notifications plugin. There is currently a known conflict with buzzer if the module is placed in Slot D, although other slots should work.
|
||||
|
||||
Further information on the RAK18001 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK18001/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="IO">
|
||||
|
||||
The [RAK13002 IO Module](https://store.rakwireless.com/collections/wisblock-interface/products/adapter-module-rak13002) can be used to, among other things, add a user button to the RAK base boards (excluding the RAK19003 Mini base board). It features a number of different interface options:
|
||||
|
||||
- 2x I<sup>2</sup>C interfaces
|
||||
- 2x UART interfaces
|
||||
- 1x SPI interface
|
||||
- Upto 6x GPIOs
|
||||
- 2x ADC interfaces
|
||||
- 3.3v Power rails
|
||||
|
||||
|
||||
There is development activity in progress to get sensors such as this added to the Meshtastic Core.
|
||||
Further information on the RAK13002 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK13002/Overview).
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="BME680">
|
||||
|
||||
The [RAK1906 Environmental Sensor](https://store.rakwireless.com/products/rak1906-bme680-environment-sensor) is based on the Bosch BME680 module and has the following features:
|
||||
|
||||
- Temperature measurement (Range -40°C to +85°C)
|
||||
- Humidity measurement (Range 0% to 100%)
|
||||
- Barometer measurement (Range 300 to 1100 hPa)
|
||||
- Air Quality measurement
|
||||
|
||||
|
||||
There is development activity in progress to get sensors such as this added to the Meshtastic Core.
|
||||
Further information on the RAK1906 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK1906/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
58
docs/hardware/devices/rak/screens.mdx
Normal file
58
docs/hardware/devices/rak/screens.mdx
Normal file
|
@ -0,0 +1,58 @@
|
|||
---
|
||||
id: screens
|
||||
title: Screens
|
||||
sidebar_label: Screens
|
||||
sidebar_position: 2
|
||||
---
|
||||
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
There are currently two different screens supported by the RAK WisBlock system:
|
||||
|
||||
<Tabs
|
||||
groupId="rakscreens"
|
||||
defaultValue="OLED"
|
||||
values={[
|
||||
{label: 'OLED Display', value: 'OLED'},
|
||||
{label: 'E-Ink Display', value: 'E-Ink'}
|
||||
]}>
|
||||
<TabItem value="OLED">
|
||||
|
||||
The [RAK1921 OLED display](https://store.rakwireless.com/products/rak1921-oled-display-panel) is a 0.96 inch monochrome display.
|
||||
|
||||
- 0.96 inch OLED display
|
||||
- Resolution 128 x 64 pixels
|
||||
- I<sup>2</sup>C interface
|
||||
|
||||
|
||||
This item requires soldering.
|
||||
Further information on the RAK1921 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK1921/Overview/#product-description).
|
||||
Similar modules are widely available from other suppliers, but do check the boards as some have the VDD and GND pins swapped round.
|
||||
|
||||
[<img alt="0.96 inch OLED display" src="/img/hardware/screen.png" style={{zoom:'25%'}} />](/img/hardware/screen.png)
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="E-Ink">
|
||||
|
||||
The [RAK1400 EPD module](https://store.rakwireless.com/products/wisblock-epd-module-rak14000) is an ultra low power E-Ink display with three user buttons.
|
||||
|
||||
- 2.13 inch black and white E-Ink display
|
||||
- Three button module
|
||||
- Resolution 212 x 104 pixels
|
||||
- Occupies the IO Port of a Wisblock Base
|
||||
|
||||
|
||||
- Firmware for 5005 with RAK14000 e-paper: [`firmware-rak4631_eink-1.3.x.uf2`](/downloads)
|
||||
|
||||
<img
|
||||
alt="RAK4631 5005 14000"
|
||||
src="/img/hardware/rak4631_5005_epaper.jpg"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
Please note only the white-black display is supported at this time, the white-black-red display may work, but is not supported.
|
||||
Further information on the RAK14000 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK14000/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
|
@ -1,339 +0,0 @@
|
|||
---
|
||||
id: wisBlock
|
||||
title: RAK WisBlock
|
||||
sidebar_label: RAK WisBlock
|
||||
sidebar_position: 1
|
||||
---
|
||||
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
The RAK WisBlock is a low power modular hardware system that can be used to build Meshtastic devices. Soldering is only required for the optional OLED screen.
|
||||
|
||||
RAK Wireless currently sell a [Meshtastic Starter kit](https://store.rakwireless.com/products/wisblock-meshtastic-starter-kit) that has the minimum you need to get started. If you wish to purchase parts separately, you will need a [base board](https://store.rakwireless.com/collections/wisblock-base), a [core 4631 LPWAN module](https://store.rakwireless.com/collections/wisblock-core/products/rak4631-lpwan-node), and optionally a GPS Sensor, screen or other module to build a Meshtastic device. Please ensure you choose the correct operating frequency for your country when purchasing.
|
||||
|
||||
Please see the RAK documentation for the correct way to connect your hardware to ensure that you do not damage the device. There is currently no pin required to pair RAK devices via BLE.
|
||||
|
||||
## RAK base boards
|
||||
<Tabs
|
||||
groupId="rakbase board"
|
||||
defaultValue="RAK5005-O"
|
||||
values={[
|
||||
{label: 'RAK5005-O', value: 'RAK5005-O'},
|
||||
{label: 'RAK19003', value: 'RAK19003'},
|
||||
{label: 'RAK19001', value: 'RAK19001'},
|
||||
{label: 'RAK19007', value:'RAK19007'}
|
||||
]}>
|
||||
<TabItem value="RAK5005-O">
|
||||
|
||||
[RAK5005-O](https://store.rakwireless.com/products/rak5005-o-base-board) - The original WisBlock Base Board
|
||||
|
||||
- Reset button
|
||||
- OLED screen support (OLED screen sold separately)
|
||||
- Connector for 3.7v LiPo battery (with charge controller)
|
||||
- Connector for 5v solar panel (max 5.5v)
|
||||
- 1x Core Module slot
|
||||
- 1x WisBlock IO Module slot
|
||||
- 4x WisBlock Sensor Module slots
|
||||
- I<sup>2</sup>C, UART, GPIOs and analog input accessible with solder contacts
|
||||
- Micro USB port for debugging and power
|
||||
|
||||
Further information on the RAK5005-O can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK5005-O/Overview/#product-description).
|
||||
|
||||
It may be possible to add a user button using the [13002 IO module](https://store.rakwireless.com/collections/wisblock-interface/products/adapter-module-rak13002).
|
||||
|
||||
- [Update the bootloader](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK4631/Quickstart/#updating-the-bootloader) on first use! This can be done easily with [Meshtastic-flasher](https://github.com/meshtastic/Meshtastic-gui-installer).
|
||||
- Firmware for 5005 base board: [`firmware-rak4631-1.x.x.uf2`](/downloads)
|
||||
|
||||
<img
|
||||
alt="RAK4631 5005"
|
||||
src="/img/hardware/rak4631_5005.png"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="RAK19003">
|
||||
|
||||
[RAK19003](https://store.rakwireless.com/products/wisblock-base-board-rak19003) - WisBlock Mini Base Board
|
||||
|
||||
- Reset button
|
||||
- OLED screen support (OLED screen sold separately)
|
||||
- Connector for 3.7v LiPo battery (with charge controller)
|
||||
- Connector for 5v solar panel (max 5.5v)
|
||||
- 1x Core Module slot
|
||||
- 2x WisBlock Sensor Module slots
|
||||
- I<sup>2</sup>C, UART and BOOT headers accessible with solder contacts
|
||||
- Micro USB port for debugging and power
|
||||
|
||||
|
||||
Further information on the RAK19003 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK19003/Overview/#product-description).
|
||||
|
||||
It is currently not possible to add a user button to this board.
|
||||
|
||||
- [Update the bootloader](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK4631/Quickstart/#updating-the-bootloader) on first use! This can be done easily with [Meshtastic-flasher](https://github.com/meshtastic/Meshtastic-gui-installer).
|
||||
- Firmware for 19003 base board: [`firmware-rak4631-1.x.x.uf2`](/downloads)
|
||||
|
||||
|
||||
<img
|
||||
alt="RAK4631 19003"
|
||||
src="/img/hardware/rak4631_19003.png"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="RAK19001">
|
||||
|
||||
[RAK19001](https://store.rakwireless.com/products/rak19001-wisblock-dual-io-base-board) - WisBlock Dual IO Base Board
|
||||
|
||||
:::caution
|
||||
This board should work with the RAK5005-O firmware, however this is currently unconfirmed.
|
||||
:::
|
||||
|
||||
- Reset and user definable buttons
|
||||
- OLED screen support (OLED screen sold separately)
|
||||
- Connector for 3.7v LiPo battery (with charge controller)
|
||||
- Separate connector for non-rechargeable batteries
|
||||
- Connector for 5v solar panel (max 5.5v)
|
||||
- 1x Core Module slot
|
||||
- 2x WisBlock IO Module slot
|
||||
- 6x WisBlock Sensor Module slots
|
||||
- I<sup>2</sup>C, SPI, UART, BOOT and GPIOs accessible with solder contacts
|
||||
- USB-C port for debugging and power
|
||||
- Battery selector switch
|
||||
|
||||
|
||||
Further information on the RAK19001 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK19001/Overview/#product-description).
|
||||
|
||||
It may be possible to add a user button using the [13002 IO module](https://store.rakwireless.com/collections/wisblock-interface/products/adapter-module-rak13002).
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="RAK19007">
|
||||
|
||||
[RAK19007](https://store.rakwireless.com/products/rak19007-wisblock-base-board-2nd-gen) - WisBlock Base Board - 2nd Generation
|
||||
This is an upgrade to the original RAK5005-O base board.
|
||||
|
||||
:::caution
|
||||
This board should work with the RAK5005-O firmware, however this is currently unconfirmed.
|
||||
:::
|
||||
|
||||
- Reset button
|
||||
- OLED screen support (OLED screen sold separately)
|
||||
- Connector for 3.7v LiPo battery (with charge controller)
|
||||
- Connector for 5v solar panel (max 5.5v)
|
||||
- 1x Core Module slot
|
||||
- 1x WisBlock IO Module slot
|
||||
- 4x WisBlock Sensor Module slots
|
||||
- I<sup>2</sup>C, UART, BOOT and GPIOs accessible with solder contacts
|
||||
- USB-C port for debugging and power
|
||||
|
||||
|
||||
Further information on the RAK19007 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK19007/Overview/#product-description).
|
||||
|
||||
It may be possible to add a user button using the [13002 IO module](https://store.rakwireless.com/collections/wisblock-interface/products/adapter-module-rak13002).
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
||||
|
||||
Flashing the firmware is a simple process. Connect your device to your computer via USB, click the reset button twice, and a drive will appear. On Windows, Linux, or Mac, drag the appropriate .uf2 firmware file onto the root of the drive and the device will restart and the firmware will be updated. Or, you can use [Meshtastic-flasher](https://github.com/meshtastic/Meshtastic-gui-installer). RAK have also provided [Installation instructions](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK4631/Quickstart/#rak4631-lora-mesh-via-meshtastic) for Meshtastic.
|
||||
|
||||
|
||||
## Core Module
|
||||
|
||||
There are two core modules supported by Meshtastic:
|
||||
|
||||
<Tabs
|
||||
groupId="rakcore"
|
||||
defaultValue="RAK4631"
|
||||
values={[
|
||||
{label: 'RAK4631', value: 'RAK4631'},
|
||||
{label: 'RAK11200', value: 'RAK11200'}
|
||||
]}>
|
||||
<TabItem value="RAK4631">
|
||||
|
||||
The main supported code is the [WisBlock LPWAN Module RAK4631](https://store.rakwireless.com/products/rak4631-lpwan-node).
|
||||
(Please note this is different to the RAK4631-R which uses different firmware incompatible with Meshtastic).
|
||||
|
||||
- nRF52840 microprocessor - Bluetooth BLE 5.0 and very low power consumption
|
||||
- SX1262 - LoRa transceiver
|
||||
- Frequency options:
|
||||
- 433 MHz
|
||||
- 470 MHz
|
||||
- 799 MHz
|
||||
- 865 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 920 MHz
|
||||
- 923 MHz
|
||||
- U.FL antenna connector
|
||||
|
||||
|
||||
<img
|
||||
alt="RAK4631 Core Module"
|
||||
src="/img/hardware/rak4631.png"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
Further information on the RAK4631 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK4631/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="RAK11200">
|
||||
|
||||
The [RAK11200](https://store.rakwireless.com/products/wiscore-esp32-module-rak11200) is currently only supported on the RAK5005-O base board. This provides the benefits of WiFi, but sacrifices the very low power consumption of the nRF microprocessor used on the RAK4631.
|
||||
|
||||
- ESP32-WROVER microprocessor - Bluetooth 4.2 & WiFi 802.11 b/g/n
|
||||
|
||||
Further information on the RAK11200 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK11200/Overview/#product-description).
|
||||
|
||||
The RAK11200 does not contain a LoRa transceiver, and this needs to be added separately in the form of the [RAK13300 LPWAN module](https://store.rakwireless.com/products/rak13300-wisblock-lpwan). This occupies the IO Port of the base board.
|
||||
|
||||
The RAK13300 has the following specifications:
|
||||
- SX1262 - LoRa transceiver
|
||||
- Frequency options:
|
||||
- 433 MHz
|
||||
- 470 MHz
|
||||
- 864 MHz
|
||||
- 865 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 920 MHz
|
||||
- 923 MHz
|
||||
- U.FL antenna connector
|
||||
|
||||
|
||||
Further information on the RAK13300 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK13300/Overview/#product-description).
|
||||
|
||||
- Firmware for 5005 with 11200: [`firmware-11200-1.3.x.bin`](/downloads)
|
||||
|
||||
<img
|
||||
alt="RAK4631 5005 11200"
|
||||
src="/img/hardware/rak11200.jpg"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
||||
|
||||
## Screens
|
||||
|
||||
There are currently two different screens supported by the RAK WisBlock system:
|
||||
|
||||
<Tabs
|
||||
groupId="rakscreens"
|
||||
defaultValue="OLED"
|
||||
values={[
|
||||
{label: 'OLED Display', value: 'OLED'},
|
||||
{label: 'E-Ink Display', value: 'E-Ink'}
|
||||
]}>
|
||||
<TabItem value="OLED">
|
||||
|
||||
The [RAK1921 OLED display](https://store.rakwireless.com/products/rak1921-oled-display-panel) is a 0.96 inch monochrome display.
|
||||
|
||||
- 0.96 inch OLED display
|
||||
- Resolution 128 x 64 pixels
|
||||
- I<sup>2</sup>C interface
|
||||
|
||||
|
||||
This item requires soldering.
|
||||
Further information on the RAK1921 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK1921/Overview/#product-description).
|
||||
Similar modules are widely available from other suppliers, but do check the boards as some have the VDD and GND pins swapped round.
|
||||
|
||||
[<img alt="0.96 inch OLED display" src="/img/hardware/screen.png" style={{zoom:'25%'}} />](/img/hardware/screen.png)
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="E-Ink">
|
||||
|
||||
The [RAK1400 EPD module](https://store.rakwireless.com/products/wisblock-epd-module-rak14000) is an ultra low power E-Ink display with three user buttons.
|
||||
|
||||
- 2.13 inch black and white E-Ink display
|
||||
- Three button module
|
||||
- Resolution 212 x 104 pixels
|
||||
- Occupies the IO Port of a Wisblock Base
|
||||
|
||||
|
||||
- Firmware for 5005 with RAK14000 e-paper: [`firmware-rak4631_eink-1.3.x.uf2`](/downloads)
|
||||
|
||||
<img
|
||||
alt="RAK4631 5005 14000"
|
||||
src="/img/hardware/rak4631_5005_epaper.jpg"
|
||||
style={{ zoom: '50%' }}
|
||||
/>
|
||||
|
||||
Please note only the white-black display is supported at this time, the white-black-red display may work, but is not supported.
|
||||
Further information on the RAK14000 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK14000/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
||||
|
||||
## Supported modules
|
||||
|
||||
<Tabs
|
||||
groupId="rakmodules"
|
||||
defaultValue="GPS"
|
||||
values={[
|
||||
{label: 'GPS Module', value: 'GPS'},
|
||||
{label: 'Buzzer', value: 'Buzzer'},
|
||||
{label: 'I/O Module', value: 'IO'},
|
||||
{label: 'Environmental Sensor', value:'BME680'}
|
||||
]}>
|
||||
<TabItem value="GPS">
|
||||
|
||||
To add a GPS to the RAK5005-O base board, you need the [RAK1910 GPS sensor](https://store.rakwireless.com/collections/wisblock-sensor/products/rak1910-max-7q-gnss-location-sensor). It is supported on slot A of the 5005 board via UART.
|
||||
|
||||
- uBlox MAX-7Q GPS module
|
||||
- GPS and GLONASS satellite support
|
||||
|
||||
|
||||
Further information on the RAK1910 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK1910/Overview/#product-description).
|
||||
|
||||
To add a GPS to the RAK19003 base board, you need the [RAK12500 GPS sensor](https://store.rakwireless.com/products/wisblock-gnss-location-module-rak12500). It is supported via I<sup>2</sup>C on slot B for firmware versions 1.49 and above.
|
||||
|
||||
- uBlox Zoe-M8Q GNSS receiver
|
||||
- GPS, GLONASS, QZSS and BeiDou satellite support
|
||||
|
||||
|
||||
Further information on the RAK12500 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK12500/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="Buzzer">
|
||||
|
||||
The [RAK18001 Buzzer Module](https://store.rakwireless.com/products/wisblock-buzzer-module-rak18001) is currently being tested for integration with the External Notifications plugin. There is currently a known conflict with buzzer if the module is placed in Slot D, although other slots should work.
|
||||
|
||||
Further information on the RAK18001 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK18001/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="IO">
|
||||
|
||||
The [RAK13002 IO Module](https://store.rakwireless.com/collections/wisblock-interface/products/adapter-module-rak13002) can be used to, among other things, add a user button to the RAK base boards (excluding the RAK19003 Mini base board). It features a number of different interface options:
|
||||
|
||||
- 2x I<sup>2</sup>C interfaces
|
||||
- 2x UART interfaces
|
||||
- 1x SPI interface
|
||||
- Upto 6x GPIOs
|
||||
- 2x ADC interfaces
|
||||
- 3.3v Power rails
|
||||
|
||||
|
||||
There is development activity in progress to get sensors such as this added to the Meshtastic Core.
|
||||
Further information on the RAK13002 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK13002/Overview).
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="BME680">
|
||||
|
||||
The [RAK1906 Environmental Sensor](https://store.rakwireless.com/products/rak1906-bme680-environment-sensor) is based on the Bosch BME680 module and has the following features:
|
||||
|
||||
- Temperature measurement (Range -40°C to +85°C)
|
||||
- Humidity measurement (Range 0% to 100%)
|
||||
- Barometer measurement (Range 300 to 1100 hPa)
|
||||
- Air Quality measurement
|
||||
|
||||
|
||||
There is development activity in progress to get sensors such as this added to the Meshtastic Core.
|
||||
Further information on the RAK1906 can be found on the [RAK Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock/RAK1906/Overview/#product-description).
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
||||
|
||||
## RAK Wireless' Resources
|
||||
|
||||
- RAK's Wisblock [Documentation Center](https://docs.rakwireless.com/Product-Categories/WisBlock)
|
||||
- RAK's [GitHub Page](https://github.com/RAKWireless/WisBlock) for the WisBlock
|
|
@ -1,32 +0,0 @@
|
|||
---
|
||||
id: station-g1
|
||||
title: Station G1 device
|
||||
sidebar_label: Station G1
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
The Station G1 is the first dedicated hardware to be designed from scratch purely for Meshtastic Licensed (HAM) Operation by Neil Hao. It has been designed to be small and compact with the inclusion of 35dBm high power PA.
|
||||
|
||||
Only the US 915 MHz version is available currently. There should be an EU 433 MHz version available in the future.
|
||||
|
||||
### Features
|
||||
|
||||
- Meshtastic pre-installed
|
||||
- ESP32 WROOM microprocessor - WiFi & Bluetooth
|
||||
- Semtech SX1262 - LoRa Transceiver
|
||||
- Frequency options:
|
||||
- 915 MHz
|
||||
- Additional 35dBm Lora Power Amplifier to boost the transmit power
|
||||
- User button
|
||||
- 1.3 inch OLED screen
|
||||
- Optional GPS Module and IO Extension Socket
|
||||
- Optional 12V Battery Docker which can be used as Backup Power, or in scenarios that require mobility
|
||||
|
||||
### Resources
|
||||
|
||||
- Firmware file: `firmware-station-g1-1.x.x.bin`
|
||||
- [Purchase link](https://www.tindie.com/products/neilhao/meshtastic-mesh-device-station-edition/)
|
||||
|
||||
[<img alt="Station G1" src="https://uniteng.com/wiki/lib/exe/fetch.php?media=meshtastic:meshtastic_mesh_device_station_edition_overview.jpg" style={{zoom:'25%'}} />](https://uniteng.com/wiki/lib/exe/fetch.php?media=meshtastic:meshtastic_mesh_device_station_edition_overview.jpg)
|
||||
|
||||
Further information on the Station G1 can be found on [Unit Engineering's Wiki](https://uniteng.com/wiki/doku.php?id=meshtastic:station).
|
14
docs/hardware/devices/station-g1/buttons.mdx
Normal file
14
docs/hardware/devices/station-g1/buttons.mdx
Normal file
|
@ -0,0 +1,14 @@
|
|||
---
|
||||
id: buttons
|
||||
title: Buttons
|
||||
sidebar_label: Buttons
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
## Functionality
|
||||
|
||||
- **Program Button**
|
||||
- _**Single press**_ changes the page of information displayed on the screen.
|
||||
- _**Double press**_ sets the Bluetooth pairing code to 123456.
|
||||
- _**Long press**_ adjusts the contrast of the screen.
|
||||
- _**Long press during reboot**_ turns on the software WiFi access point.
|
43
docs/hardware/devices/station-g1/index.mdx
Normal file
43
docs/hardware/devices/station-g1/index.mdx
Normal file
|
@ -0,0 +1,43 @@
|
|||
---
|
||||
id: station-g1
|
||||
title: Station G1 device
|
||||
sidebar_label: Station G1
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
The Station G1 is the second dedicated hardware device to be designed from scratch purely for Meshtastic Licensed (HAM) Operation by Neil Hao. It has been designed to be small and compact with the inclusion of 35dBm high power PA.
|
||||
|
||||
### Specifications
|
||||
|
||||
- **MCU**
|
||||
- ESP32 WROOM (WiFi & Bluetooth)
|
||||
- Bluetooth 4.2
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX1262
|
||||
- Additional 35dBm LoRa Power Amplifier to boost transmit power
|
||||
- **Frequency options**
|
||||
- US-915 MHz
|
||||
- EU-868 MHz
|
||||
- **Navigation Module**
|
||||
- ATGM336H-5N-71 (Supports GPS, BDS and GLONASS)
|
||||
- **Antenna**
|
||||
- SMA Socket
|
||||
- **Connectors**
|
||||
- USB-C
|
||||
|
||||
### Features
|
||||
|
||||
- Meshtastic pre-installed
|
||||
- User button
|
||||
- 1.3 inch OLED screen
|
||||
- Optional GPS Module and IO Extension Socket
|
||||
- Optional [12V Battery Docker](https://shop.uniteng.com/product/12v-battery-docker-for-station-edition-g1/) which can be used as Backup Power, or in scenarios that require mobility
|
||||
|
||||
### Resources
|
||||
|
||||
- Firmware file: `firmware-station-g1-1.x.x.bin`
|
||||
- [Purchase link](https://www.tindie.com/products/neilhao/meshtastic-mesh-device-station-edition/)
|
||||
|
||||
Further information on the Station G1 can be found on [Unit Engineering's Wiki](https://uniteng.com/wiki/doku.php?id=meshtastic:station).
|
||||
|
||||
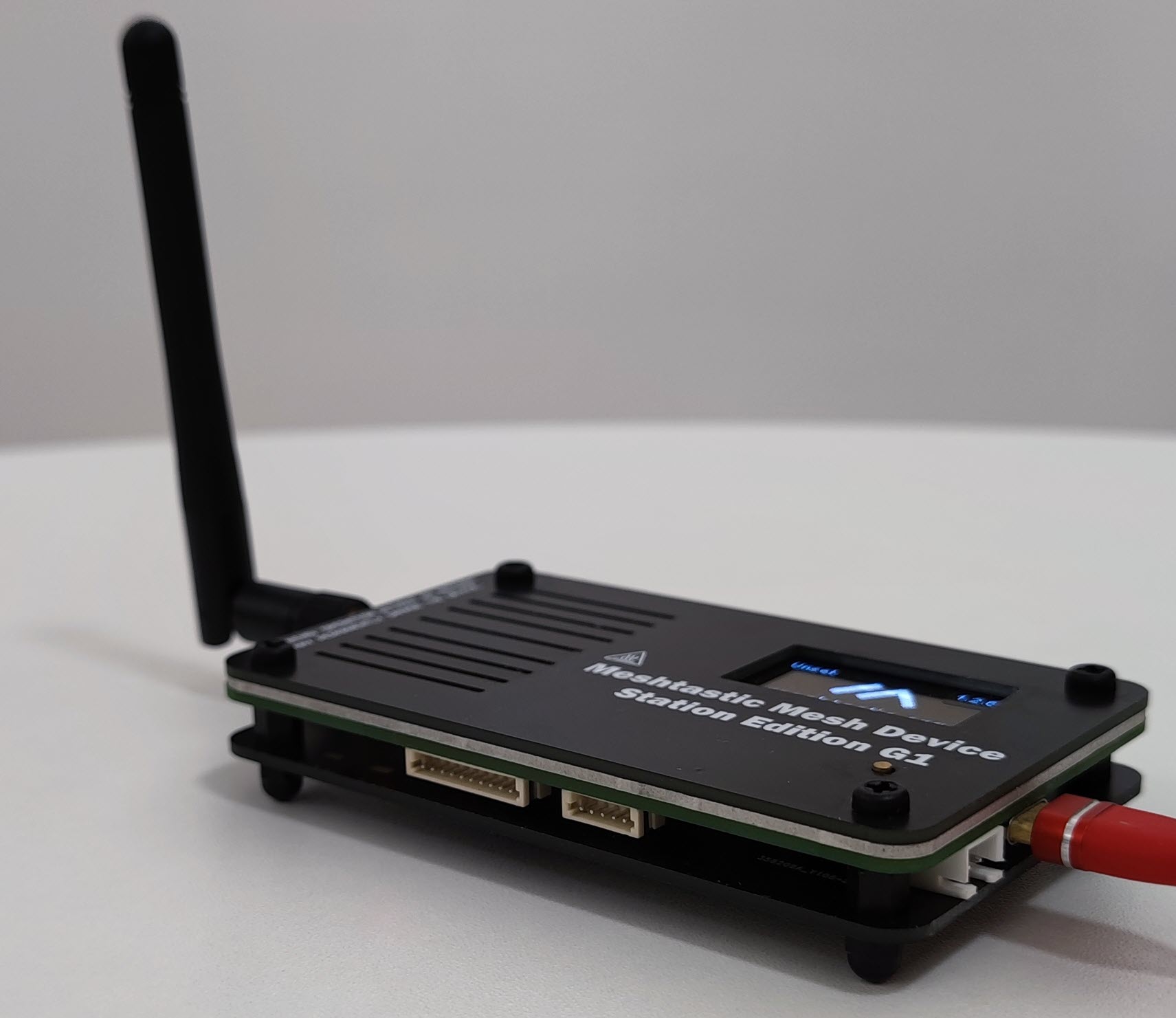
|
|
@ -1,138 +0,0 @@
|
|||
---
|
||||
id: tbeam
|
||||
title: LILYGO® TTGO T-Beam devices
|
||||
sidebar_label: LILYGO® T-Beam
|
||||
sidebar_position: 5
|
||||
---
|
||||
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
There are several versions of the T-Beam
|
||||
|
||||
<Tabs
|
||||
groupId="t-beam"
|
||||
defaultValue="1.1"
|
||||
values={[
|
||||
{label: 'T-Beam v1.1', value: '1.1'},
|
||||
{label: 'T-Beam with M8N', value: 'm8n'},
|
||||
{label: 'T-Beam with M8N & SX1262', value: 'sx1262'},
|
||||
{label: 'T-Beam v0.7', value:'0.7'}
|
||||
]}>
|
||||
|
||||
<TabItem value="1.1">
|
||||
|
||||
- Meshtastic preinstalled
|
||||
- ESP32 - WiFi & Bluetooth
|
||||
- SX1276 - LoRa Transceiver
|
||||
- Frequency options:
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 923 MHz
|
||||
- NEO-6M - GPS receiver
|
||||
- SMA antenna connector
|
||||
- Power, Program and Reset switches
|
||||
- **Comes with 0.96 inch OLED display (some soldering required to assemble)**
|
||||
|
||||
|
||||
- Firmware file: `firmware-tbeam-1.x.x.bin`
|
||||
- [Purchase link](https://www.aliexpress.com/item/4001178678568.html)
|
||||
|
||||
[<img alt="LILYGO® TTGO T-Beam Meshtastic" src="/img/hardware/t-beam-meshtastic.png" style={{zoom:'25%'}} />](/img/hardware/t-beam-meshtastic.png)
|
||||
<!--[<img alt="LILYGO® TTGO T-Beam v1.1" src="/img/hardware/t-beam-v1.1.png" style={{zoom:'35%'}} />](/img/hardware/t-beam-v1.1.png)-->
|
||||
[<img alt="LILYGO® TTGO T-Beam v1.1 pinmap" src="/img/hardware/t-beam_v1.1_pinmap.webp" style={{zoom:'35%'}} />](/img/hardware/t-beam_v1.1_pinmap.webp)
|
||||
|
||||
</TabItem>
|
||||
|
||||
<TabItem value="m8n">
|
||||
|
||||
- ESP32 - WiFi & Bluetooth
|
||||
- SX1276 - LoRa Transceiver
|
||||
- Frequency options:
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 923 MHz
|
||||
- **NEO-M8N - GNSS receiver (supports GPS, GLONASS, Galileo, BeiDou) - better GPS sensitivity**
|
||||
- U.FL antenna connector
|
||||
- Power, Program and Reset switches
|
||||
- Screen sold separately
|
||||
|
||||
|
||||
- Firmware file: `firmware-tbeam-1.x.x.bin`
|
||||
- [Purchase link](https://www.aliexpress.com/item/33047631119.html)
|
||||
|
||||
[<img alt="LILYGO® TTGO T-Beam M8N" src="/img/hardware/t-beam-m8n.png" style={{zoom:'25%'}} />](/img/hardware/t-beam-m8n.png)
|
||||
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="sx1262">
|
||||
|
||||
- ESP32 - WiFi & Bluetooth
|
||||
- **SX1262 - LoRa Transceiver - improved performance**
|
||||
- Frequency options:
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 923 MHz
|
||||
- **NEO-M8N - GNSS receiver (supports GPS, GLONASS, Galileo, BeiDou) - better GPS sensitivity**
|
||||
- U.FL antenna connector
|
||||
- Power, Program and Reset switches
|
||||
- Screen sold separately
|
||||
|
||||
|
||||
- Firmware file: `firmware-tbeam-1.x.x.bin`
|
||||
- [Purchase link](https://www.aliexpress.com/item/4001287221970.html)
|
||||
|
||||
[<img alt="LILYGO® TTGO T-Beam M8N & SX1262" src="/img/hardware/t-beam-sx1262.png" style={{zoom:'25%'}} />](/img/hardware/t-beam-sx1262.png)
|
||||
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="0.7">
|
||||
|
||||
:::note
|
||||
This is an earlier version of the T-Beam board, and due to changes in the design in subsequent iterations this board uses a specific firmware file different from the other T-Beam boards.
|
||||
|
||||
`firmware-tbeam0.7-1.x.x.bin` is the correct firmware. `firmware-tbeam-1.x.x.bin` is incompatible. For all other T-Beam boards `firmware-tbeam-1.x.x.bin` is the correct selection.
|
||||
:::
|
||||
|
||||
- ESP32 - WiFi & Bluetooth
|
||||
- SX1276 - LoRa Transceiver
|
||||
- Frequency options:
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- NEO-6M - GPS receiver
|
||||
- SMA antenna connector
|
||||
- Power, Program and Reset switches
|
||||
- No GPS
|
||||
- Screen sold separately
|
||||
|
||||
|
||||
- Firmware file: `firmware-tbeam0.7-1.x.x.bin`
|
||||
- [Purchase link](https://www.aliexpress.com/item/4000469332610.html)
|
||||
|
||||
[<img alt="LILYGO TTGO T-Beam v0.7" src="/img/hardware/t-beam-v0.7.png" style={{zoom:'25%'}} />](/img/hardware/t-beam-v0.7.png)
|
||||
[<img alt="LILYGO TTGO T-Beam v0.7 pinmap" src="/img/hardware/t-beam_v0.7_pinmap.jpeg" style={{zoom:'25%'}} />](/img/hardware/t-beam_v0.7_pinmap.jpeg)
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
||||
|
||||
Further information on the LILYGO® T-Beam and LoRa devices can be found on LILYGO®'s [GitHub page](https://github.com/Xinyuan-LilyGO/LilyGo-LoRa-Series).
|
||||
|
||||
:::note
|
||||
All T-beam models have an 18650 size battery holder on the rear of the device. This is designed to the original specification of the 18650, and only fits unprotected flat top 18650 cells. Button top and protected cells are typically longer than 650mm, often approaching 700mm.
|
||||
:::
|
||||
|
||||
## Screen
|
||||
|
||||
- 0.96 inch OLED I<sup>2</sup>C display
|
||||
- [Purchase link](https://www.aliexpress.com/item/32922106384.html)
|
||||
|
||||
|
||||
[<img alt="0.96 inch OLED display" src="/img/hardware/screen.png" style={{zoom:'25%'}} />](/img/hardware/screen.png)
|
||||
|
||||
To attach the screen, connect VCC to 3v3, GND to GND, SCL to pin 22, and SDA to pin 21 as per the below image.
|
||||
|
||||
[<img alt="Connecting the OLED screen to a T-Beam" src="/img/hardware/t-beam-screen.jpg" style={{zoom:'50%'}} />](/img/hardware/t-beam-screen.jpg)
|
18
docs/hardware/devices/tbeam/buttons.mdx
Normal file
18
docs/hardware/devices/tbeam/buttons.mdx
Normal file
|
@ -0,0 +1,18 @@
|
|||
---
|
||||
id: buttons
|
||||
title: Buttons
|
||||
sidebar_label: Buttons
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
## Functionality
|
||||
|
||||
- **Reset Button**
|
||||
- _**Single press**_ resets the device.
|
||||
- **Power Button**
|
||||
- _**Long press**_ powers the device off or turns it back on again.
|
||||
- **Program button**
|
||||
- _**Single press**_ changes the page of information displayed on the screen.
|
||||
- _**Double press**_ sets the Bluetooth pairing code to `123456` (useful if you do not have a screen on the device).
|
||||
- _**Long press**_ adjusts the contrast of the screen.
|
||||
- _**Long press during reboot**_ turns on the software WiFi access point.
|
24
docs/hardware/devices/tbeam/enclosures.mdx
Normal file
24
docs/hardware/devices/tbeam/enclosures.mdx
Normal file
|
@ -0,0 +1,24 @@
|
|||
---
|
||||
id: enclosures
|
||||
title: Enclosures
|
||||
sidebar_label: Enclosures
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
import TrophoTbeam from '/img/enclosures/3dp-tropho-tbeam.jpg';
|
||||
|
||||
## 3D Printed
|
||||
|
||||
### Created by tropho/TonyG
|
||||
|
||||
#### TTGO T-Beam V5 Case
|
||||
|
||||
Download from [Printables](https://www.printables.com/model/127253-t-beam-case-for-meshtastic-v5) or purchase from the creator's [Etsy Store](https://www.etsy.com/listing/1173559418/meshtastic-t-beam-case-for-neo-m8n).
|
||||
|
||||
##### Required Hardware
|
||||
|
||||
- (x4) M3x16mm socket-head cap screws
|
||||
- (x4) M3 nuts
|
||||
- (x4) M2x4mm screws (no nuts) to secure T-Beam to the frame
|
||||
|
||||
<img src={TrophoTbeam} width="600" align="left" />
|
155
docs/hardware/devices/tbeam/index.mdx
Normal file
155
docs/hardware/devices/tbeam/index.mdx
Normal file
|
@ -0,0 +1,155 @@
|
|||
---
|
||||
id: tbeam
|
||||
title: LILYGO® TTGO T-Beam Devices
|
||||
sidebar_label: LILYGO® T-Beam
|
||||
sidebar_position: 5
|
||||
---
|
||||
|
||||
import Tabs from '@theme/Tabs';
|
||||
import TabItem from '@theme/TabItem';
|
||||
|
||||
All T-beam models have an 18650 size battery holder on the rear of the device. This is designed to the original specification of the 18650 and only fits unprotected flat top 18650 cells. Button top and protected cells are typically longer than 650mm, often approaching 700mm.
|
||||
|
||||
Further information on the LILYGO® T-Beam devices can be found on LILYGO®'s [GitHub page](https://github.com/Xinyuan-LilyGO/LilyGo-LoRa-Series).
|
||||
|
||||
|
||||
<Tabs
|
||||
groupId="t-beam"
|
||||
defaultValue="sx1262"
|
||||
values={[
|
||||
{label: 'T-Beam v0.7', value:'0.7'},
|
||||
{label: 'T-Beam v1.1', value: '1.1'},
|
||||
{label: 'T-Beam with M8N', value: 'm8n'},
|
||||
{label: 'T-Beam with M8N & SX1262', value: 'sx1262'}
|
||||
]}>
|
||||
|
||||
<TabItem value="0.7">
|
||||
|
||||
:::caution Firmware Version Notice
|
||||
This is an earlier version of the T-Beam board. Due to changes in the design this board uses a specific firmware file different from the other T-Beam boards.
|
||||
:::
|
||||
|
||||
|
||||
- **MCU**
|
||||
- ESP32 (WiFi & Bluetooth)
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX1276
|
||||
- **Frequency options**
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- **Navigation Module**
|
||||
- NEO-6M (GPS receiver)
|
||||
- **Connectors**
|
||||
- Micro USB
|
||||
- Antenna: SMA antenna connector
|
||||
|
||||
**Features**
|
||||
- Meshtastic preinstalled
|
||||
- Power, Program and Reset switches
|
||||
- Screen sold separately
|
||||
- No GPS
|
||||
|
||||
**Resources**
|
||||
- Firmware file: `firmware-tbeam0.7-X.X.X.xxxxxxx.bin`
|
||||
- Purchase link: [AliExpress](https://www.aliexpress.com/item/4000469332610.html)
|
||||
|
||||
|
||||

|
||||

|
||||
|
||||
</TabItem>
|
||||
<TabItem value="1.1">
|
||||
|
||||
- **MCU**
|
||||
- ESP32 (WiFi & Bluetooth)
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX1276
|
||||
- **Frequency options**
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 923 MHz
|
||||
- **Navigation Module**
|
||||
- NEO-6M (GPS receiver)
|
||||
- **Connectors**
|
||||
- Micro USB
|
||||
- Antenna: SMA antenna connector
|
||||
|
||||
**Features**
|
||||
- Meshtastic preinstalled
|
||||
- Power, Program and Reset switches
|
||||
- **Comes with 0.96 inch OLED display** (soldering required to assemble)
|
||||
|
||||
**Resources**
|
||||
- Firmware file: `firmware-tbeam-X.X.X.xxxxxxx.bin`
|
||||
- Purchase link: [AliExpress](https://www.aliexpress.com/item/4001178678568.html)
|
||||
|
||||

|
||||

|
||||
|
||||
</TabItem>
|
||||
|
||||
<TabItem value="m8n">
|
||||
|
||||
- **MCU**
|
||||
- ESP32 (WiFi & Bluetooth)
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX1276
|
||||
- **Frequency options**
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 923 MHz
|
||||
- **Navigation Module**
|
||||
- **NEO-M8N - GNSS receiver (supports GPS, GLONASS, Galileo, BeiDou)** (better GPS sensitivity)
|
||||
- **Connectors**
|
||||
- Micro USB
|
||||
- Antenna: U.FL antenna connector
|
||||
|
||||
**Features**
|
||||
- Meshtastic preinstalled
|
||||
- Power, Program and Reset switches
|
||||
- Screen sold separately
|
||||
|
||||
**Resources**
|
||||
- Firmware file: `firmware-tbeam-X.X.X.xxxxxxx.bin`
|
||||
- Purchase link: [AliExpress](https://www.aliexpress.com/item/33047631119.html)
|
||||
|
||||
[TTGO T-Beam M8N](/img/hardware/t-beam-m8n.png)
|
||||
|
||||
|
||||
</TabItem>
|
||||
<TabItem value="sx1262">
|
||||
|
||||
|
||||
- **MCU**
|
||||
- ESP32 (WiFi & Bluetooth)
|
||||
- **LoRa Transceiver**
|
||||
- **Semtech SX1262** (improved performance)
|
||||
- **Frequency options**
|
||||
- 433 MHz
|
||||
- 868 MHz
|
||||
- 915 MHz
|
||||
- 923 MHz
|
||||
- **Navigation Module**
|
||||
- **NEO-M8N - GNSS receiver (supports GPS, GLONASS, Galileo, BeiDou)** (better GPS sensitivity)
|
||||
- **Connectors**
|
||||
- Micro USB
|
||||
- Antenna: U.FL antenna connector
|
||||
|
||||
**Features**
|
||||
- Meshtastic preinstalled
|
||||
- Power, Program and Reset switches
|
||||
- Screen sold separately
|
||||
|
||||
**Resources**
|
||||
- Firmware file: `firmware-tbeam-X.X.X.xxxxxxx.bin`
|
||||
- Purchase link: [AliExpress](https://www.aliexpress.com/item/4001287221970.html)
|
||||
|
||||
|
||||
[T-Beam M8N & SX1262](/img/hardware/t-beam-sx1262.png)
|
||||
|
||||
|
||||
</TabItem>
|
||||
</Tabs>
|
24
docs/hardware/devices/tbeam/screens.mdx
Normal file
24
docs/hardware/devices/tbeam/screens.mdx
Normal file
|
@ -0,0 +1,24 @@
|
|||
---
|
||||
id: screens
|
||||
title: Screens
|
||||
sidebar_label: Screens
|
||||
sidebar_position: 2
|
||||
---
|
||||
|
||||
|
||||
## 0.96 inch OLED I<sup>2</sup>C display
|
||||
|
||||
- [Purchase link](https://www.aliexpress.com/item/32922106384.html)
|
||||
|
||||

|
||||
|
||||
### Pin map
|
||||
|
||||
To attach the screen:
|
||||
|
||||
1. Connect VCC to 3v3
|
||||
2. Connect GND to GND
|
||||
3. Connect SCL to pin 22
|
||||
4. Connect SDA to pin 21
|
||||
|
||||

|
|
@ -1,45 +0,0 @@
|
|||
---
|
||||
id: techo
|
||||
title: LILYGO® TTGO T-Echo devices
|
||||
sidebar_label: LILYGO® T-Echo
|
||||
sidebar_position: 2
|
||||
---
|
||||
|
||||
The T-Echo is the latest device to be release by LILYGO® supporting a low power consumption micro-controller.
|
||||
|
||||
### Features
|
||||
|
||||
- nRF52840 - Bluetooth BLE 5.0, NFC and very low power consumption
|
||||
- SX1262 - LoRa transceiver
|
||||
- 1.54" eInk display
|
||||
- L76K - GNSS receiver - Supporting GPS, BeiDou, GLONASS & QZSS
|
||||
- Reset, Program and capacitive touch buttons
|
||||
- U.FL antenna connector
|
||||
- Optional BME280 - Humidity and Pressure Sensor
|
||||
- Comes with a case and battery
|
||||
|
||||
|
||||
- Firmware file: `firmware-t-echo-2.x.x.uf2`
|
||||
- [Purchase link](https://www.aliexpress.com/item/1005002842456390.html)
|
||||
- TTGO's [GitHub page](https://github.com/Xinyuan-LilyGO/LilyGO-T-Echo) for the T-Echo
|
||||
|
||||
<img
|
||||
alt="LILYGO T-Echo"
|
||||
src="/img/hardware/t-echo.png"
|
||||
style={{ zoom: '15%' }}
|
||||
/>
|
||||
|
||||
### T-Echo button functions
|
||||
|
||||
- Capacitive top button
|
||||
- A short press refreshes the current screen
|
||||
- Button 1
|
||||
- A single press resets the device
|
||||
- A double press puts the device into bootloader mode ready to receive a new firmware
|
||||
- Button 2
|
||||
- A single press changes the page displayed on the device
|
||||
- A double press turns the screen backlight on/off
|
||||
- A long press turns the device off
|
||||
|
||||
|
||||
[<img alt="LILYGO T-Echo" src="/img/hardware/t-echo-lilygo.jpg" style={{zoom:'25%'}} />](/img/hardware/t-echo-lilygo.jpg)
|
21
docs/hardware/devices/techo/buttons.mdx
Normal file
21
docs/hardware/devices/techo/buttons.mdx
Normal file
|
@ -0,0 +1,21 @@
|
|||
---
|
||||
id: buttons
|
||||
title: Buttons
|
||||
sidebar_label: Buttons
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
### T-Echo button functions
|
||||
|
||||
- **Capacitive Button (Top)**
|
||||
- _Short press_ refreshes the current screen.
|
||||
- **Reset Button (Button 1)**
|
||||
- _Single press_ resets the device.
|
||||
- _Double press_ puts the device into bootloader mode ready to receive new firmware.
|
||||
- **Program/Power Button (Button 2)**
|
||||
- _Single press_ changes the page displayed on the device.
|
||||
- A double press turns the screen backlight on/off
|
||||
- A long press turns the device off
|
||||
|
||||
|
||||

|
37
docs/hardware/devices/techo/index.mdx
Normal file
37
docs/hardware/devices/techo/index.mdx
Normal file
|
@ -0,0 +1,37 @@
|
|||
---
|
||||
id: techo
|
||||
title: LILYGO® TTGO T-Echo devices
|
||||
sidebar_label: LILYGO® T-Echo
|
||||
sidebar_position: 2
|
||||
---
|
||||
|
||||
The T-Echo is the latest device to be release by LILYGO® supporting a low power consumption micro-controller.
|
||||
|
||||
### Specifications
|
||||
|
||||
- **MCU**
|
||||
- nRF52840
|
||||
- Bluetooth BLE 5.0 & NFC
|
||||
- Very low power consumption
|
||||
- **LoRa Transceiver**
|
||||
- Semtech SX1262
|
||||
- **Frequency options**
|
||||
- 915 MHz
|
||||
- **Navigation Module**
|
||||
- L76K GNSS receiver (Supports GPS, BeiDou, GLONASS & QZSS)
|
||||
- **Connectors**
|
||||
- U.FL antenna connector
|
||||
|
||||
### Features
|
||||
- Reset, Program and capacitive touch buttons
|
||||
- 1.54" eInk display
|
||||
- Optional BME280 - Humidity and Pressure Sensor
|
||||
- Comes with a case and battery
|
||||
|
||||
### Resources
|
||||
|
||||
- Firmware file: `firmware-t-echo-2.x.x.uf2`
|
||||
- [Purchase link](https://www.aliexpress.com/item/1005002842456390.html)
|
||||
- TTGO's [GitHub page](https://github.com/Xinyuan-LilyGO/LilyGO-T-Echo) for the T-Echo
|
||||
|
||||

|
|
@ -1,8 +0,0 @@
|
|||
---
|
||||
id: 3dprinted-enclosure
|
||||
title: 3D Printed Enclosures
|
||||
sidebar_label: 3D Printed
|
||||
sidebar_position: 1
|
||||
---
|
||||
|
||||
... WIP
|
|
@ -1,7 +0,0 @@
|
|||
label: Enclosures
|
||||
collapsible: true
|
||||
position: 3
|
||||
link:
|
||||
type: generated-index
|
||||
title: Enclosures
|
||||
slug: enclosures
|
|
@ -1,30 +0,0 @@
|
|||
---
|
||||
id: buttons
|
||||
title: Buttons
|
||||
sidebar_label: Buttons
|
||||
sidebar_position: 3
|
||||
---
|
||||
|
||||
## Functionality
|
||||
|
||||
A number of devices have buttons that can be used to interact with the firmware. These buttons have a number of different functions.
|
||||
|
||||
### Reset Button
|
||||
|
||||
The reset button is present on most devices.
|
||||
|
||||
- _Single press_ resets the device.
|
||||
|
||||
### Power Button
|
||||
|
||||
The power button is present on some devices.
|
||||
|
||||
- _Long press_ powers the device off or turns it back on again.
|
||||
|
||||
### Program button
|
||||
|
||||
The program button is present of some devices and has a number of functions:
|
||||
- _Single press_ changes the page of information displayed on the screen.
|
||||
- _Double press_ sets the Bluetooth pairing code to `123456` (useful if you do not have a screen on the device).
|
||||
- _Long press_ adjusts the contrast of the screen.
|
||||
- _Long press during reboot_ turns on the software WiFi access point on devices that support WiFi.
|
|
@ -1,179 +0,0 @@
|
|||
---
|
||||
id: peripherals
|
||||
title: A Note on Peripherals
|
||||
sidebar_label: Peripherals
|
||||
sidebar_position: 2
|
||||
---
|
||||
|
||||
## Firmware Versions
|
||||
|
||||
:::warning
|
||||
GPIO access is fundamentally dangerous because invalid options can physically damage or destroy your hardware. Ensure that you fully understand the schematic for your particular device before trying this as we do not offer a warranty. Use at your own risk.
|
||||
:::
|
||||
|
||||
The device firmware runs on the nodes to build the mesh for communication. Each different make and model of device requires a different build of the Meshtastic firmware in order to run properly. Thankfully, due to the design of Meshtastic, it is possible to port the firmware to new devices as they become available. The firmware currently runs on a range of ESP32 based devices, but there is also increasing support for the nRF52 microprocessor with some more recent devices coming to market.
|
||||
|
||||
The current firmware has support for a screen to display received messages, along with information about nodes on the mesh, and more detailed information about the device on which it is running.
|
||||
|
||||
The latest firmware can be downloaded from the [Downloads](/downloads) page. If you wish to view the code or contribute to development of the firmware, please visit the device code [GitHub page](https://github.com/meshtastic/firmware).
|
||||
|
||||
:::info
|
||||
Please be aware that there are significant changes between version branches 1.2.x and 1.3.x which mean that devices need to be running the same branch of firmware to be able to talk to each other. Python, Android, and other software applications will also need to be running the same branch to be able to talk to the device.
|
||||
|
||||
This feature uses a preinstalled module in the device code and associated command line flags/classes in the python code. You'll need to be running at least version 1.2.23 (or later) of the python and device code to use this feature.
|
||||
:::
|
||||
|
||||
## Remote Hardware
|
||||
|
||||
### Supported Operations
|
||||
|
||||
- Set any GPIO
|
||||
- Read any GPIO
|
||||
- Receive notification of changes in any GPIO
|
||||
|
||||
### Setup
|
||||
|
||||
You can get the latest python tool/library with `pip3 install --upgrade meshtastic` on Windows/Linux/OS-X. See the [python section](/docs/software/python/cli/installation) for more details.
|
||||
|
||||
To prevent access from untrusted users, you must first make a `gpio` channel that is used for authenticated access to this feature. You'll need to install this channel on both the local and remote node.
|
||||
|
||||
The procedure using the python command line tool is:
|
||||
|
||||
1. Connect local device via USB
|
||||
2. Create a GPIO channel:
|
||||
```shell
|
||||
meshtastic --ch-add gpio
|
||||
```
|
||||
3. If doing local testing, you may also want to change the speed of the channel:
|
||||
```sh
|
||||
meshtastic --ch-mediumfast
|
||||
```
|
||||
4. Check the channel has been created and copy the long "Complete URL" that contains all the channels on that device:
|
||||
```shell
|
||||
meshtastic --info
|
||||
```
|
||||
5. Connect the remote device via USB (or use the [remote admin](/docs/configuration/remote-admin) feature to reach it through the mesh)
|
||||
6. Set it to join the gpio channel you created:
|
||||
```shell
|
||||
meshtastic --seturl theurlyoucopiedinstep3
|
||||
```
|
||||
|
||||
Now both devices should be able to talk over the `gpio` channel. Send a text message from one the other other verify. Also run `--nodes` to verify the second node shows up.
|
||||
|
||||
### Masks
|
||||
|
||||
To determine the appropriate mask for the pin(s) that you want to know. The python program (and output) below might help:
|
||||
|
||||
```python
|
||||
>>> for i in range(1,45):
|
||||
... print(f'GPIO:{i} mask:{hex(2**i)}')
|
||||
...
|
||||
GPIO:1 mask:0x2
|
||||
GPIO:2 mask:0x4
|
||||
GPIO:3 mask:0x8
|
||||
GPIO:4 mask:0x10
|
||||
GPIO:5 mask:0x20
|
||||
GPIO:6 mask:0x40
|
||||
GPIO:7 mask:0x80
|
||||
GPIO:8 mask:0x100
|
||||
GPIO:9 mask:0x200
|
||||
GPIO:10 mask:0x400
|
||||
GPIO:11 mask:0x800
|
||||
GPIO:12 mask:0x1000
|
||||
GPIO:13 mask:0x2000
|
||||
GPIO:14 mask:0x4000
|
||||
GPIO:15 mask:0x8000
|
||||
GPIO:16 mask:0x10000
|
||||
GPIO:17 mask:0x20000
|
||||
GPIO:18 mask:0x40000
|
||||
GPIO:19 mask:0x80000
|
||||
GPIO:20 mask:0x100000
|
||||
GPIO:21 mask:0x200000
|
||||
GPIO:22 mask:0x400000
|
||||
GPIO:23 mask:0x800000
|
||||
GPIO:24 mask:0x1000000
|
||||
GPIO:25 mask:0x2000000
|
||||
GPIO:26 mask:0x4000000
|
||||
GPIO:27 mask:0x8000000
|
||||
GPIO:28 mask:0x10000000
|
||||
GPIO:29 mask:0x20000000
|
||||
GPIO:30 mask:0x40000000
|
||||
GPIO:31 mask:0x80000000
|
||||
GPIO:32 mask:0x100000000
|
||||
GPIO:33 mask:0x200000000
|
||||
GPIO:34 mask:0x400000000
|
||||
GPIO:35 mask:0x800000000
|
||||
GPIO:36 mask:0x1000000000
|
||||
GPIO:37 mask:0x2000000000
|
||||
GPIO:38 mask:0x4000000000
|
||||
GPIO:39 mask:0x8000000000
|
||||
GPIO:40 mask:0x10000000000
|
||||
GPIO:41 mask:0x20000000000
|
||||
GPIO:42 mask:0x40000000000
|
||||
GPIO:43 mask:0x80000000000
|
||||
GPIO:44 mask:0x100000000000
|
||||
```
|
||||
|
||||
## Testing GPIO Operations
|
||||
|
||||
You can programmatically do operations from your own python code by using the Meshtastic `RemoteHardwareClient` class. See the [Python API](/docs/software/python/cli/installation) documentation for more details.
|
||||
|
||||
You can add a simple LED and resistor to validate that the GPIO operations work as expected. Use [this tutorial](https://www.instructables.com/Slide-Switch-With-Arduino-Uno-R3/) as a guide.
|
||||
|
||||
### Requirements
|
||||
|
||||
- (x2) Meshtastic devices (one device could be on a local computer, and the other one just has to be powered and is the one with the LED to be connected to it)
|
||||
- (x2) wires (black and yellow; they can be any color but typically black is used for ground)
|
||||
- (x1) LED
|
||||
- (x1) 220Ω resistor (somewhat optional, but recommended)
|
||||
- (x1) Breadboard (optional)
|
||||
|
||||
### Preparation
|
||||
|
||||
1. Disconnect the remote device from power (battery/usb)
|
||||
2. Add a resistor from yellow wire to the one end of the LED (either end of the resistor is OK, either end of the LED is OK)
|
||||
3. Add the yellow wire from a GPIO pin that will not cause any issues (ex: for TLoraV1, we can use GPIO21)
|
||||
4. Add the black "ground" wire from the ground pin on the device (ex: for TLoraV1 it is the end pin next to the RST button) to the other end of the LED
|
||||
5. Power on the device
|
||||
|
||||
### Validation
|
||||
|
||||
By default, the pin may be "off" or "on". (It will most likely "off".) See the steps below for running commands. In the example of GPIO21, the mask would be `0x200000`.
|
||||
|
||||

|
||||
|
||||
|
||||
## Using GPIOs from the Python CLI
|
||||
|
||||
### Writing a GPIO
|
||||
|
||||
```shell title="Example: turning 'on' GPIO4"
|
||||
meshtastic --port /dev/ttyUSB0 --gpio-wrb 4 1 --dest 28979058
|
||||
# Connected to radio
|
||||
# Writing GPIO mask 0x10 with value 0x10 to !28979058
|
||||
```
|
||||
|
||||
### Reading a GPIO
|
||||
|
||||
```shell title="Example: read GPIO4"
|
||||
meshtastic --port /dev/ttyUSB0 --gpio-rd 0x10 --dest 28979058
|
||||
# Connected to radio
|
||||
# Reading GPIO mask 0x10 from !28979058
|
||||
# GPIO read response gpio_value=16
|
||||
```
|
||||
|
||||
:::note
|
||||
If the mask and the gpio_value match, then the value is "on". If the gpio_value is 0, then the value is "off".
|
||||
:::
|
||||
|
||||
### Watching for GPIO Changes
|
||||
|
||||
```shell title="Example: watching GPIO4 for changes"
|
||||
meshtastic --port /dev/ttyUSB0 --gpio-watch 0x10 --dest 28979058
|
||||
# Connected to radio
|
||||
# Watching GPIO mask 0x10 from !28979058
|
||||
# Received RemoteHardware typ=GPIOS_CHANGED, gpio_value=16
|
||||
# Received RemoteHardware typ=GPIOS_CHANGED, gpio_value=0
|
||||
# Received RemoteHardware typ=GPIOS_CHANGED, gpio_value=16
|
||||
# < press ctrl-c to exit >
|
||||
```
|
|
@ -1,32 +0,0 @@
|
|||
---
|
||||
id: screens
|
||||
title: Screens
|
||||
sidebar_label: Screens
|
||||
sidebar_position: 2
|
||||
---
|
||||
|
||||
A number of devices have screens capable of displaying the messages received, information about the mesh, and other details. On powering the device it will display the Meshtastic splash screen with the version number for a couple of seconds:
|
||||
|
||||

|
||||
|
||||
The screen is split up into pages, through which you can navigate using the program button as described above. The first page to be displayed will be the message screen where received messages are displayed along with the name of the node it came from. The devices will automatically switch to this page when a new message is received.
|
||||
|
||||

|
||||
|
||||
The next pages display information about the nodes that are currently on the mesh. This includes the distance and direction to that node, the signal strength, and the time last seen.
|
||||
|
||||
 
|
||||
|
||||
The next page shows information about the device, battery power, current / total nodes, number of GPS satellites seen, channel name, last digits of the MAC address, and a brief log including the names of the last nodes to join the mesh.
|
||||
|
||||

|
||||
|
||||
The final page shows current battery voltage and percent charge, as well as noting how long the device has been online and the current GPS time, and GPS location.
|
||||
|
||||

|
||||
|
||||
If the device WiFi has been enabled (only possible on ESP32 devices), another page appears displaying information about the WiFi settings, IP address, and number of devices connected to the WiFi.
|
||||
|
||||

|
||||
|
||||
With a further press of the program button, the screen will cycle round to the message page.
|
BIN
static/img/enclosures/3dp-tropho-lora32.png
Normal file
BIN
static/img/enclosures/3dp-tropho-lora32.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 432 KiB |
BIN
static/img/enclosures/3dp-tropho-rak19003-micro.png
Normal file
BIN
static/img/enclosures/3dp-tropho-rak19003-micro.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 532 KiB |
BIN
static/img/enclosures/3dp-tropho-rak19003.png
Normal file
BIN
static/img/enclosures/3dp-tropho-rak19003.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 282 KiB |
BIN
static/img/enclosures/3dp-tropho-rak19007.png
Normal file
BIN
static/img/enclosures/3dp-tropho-rak19007.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 274 KiB |
BIN
static/img/enclosures/3dp-tropho-rak5005.png
Normal file
BIN
static/img/enclosures/3dp-tropho-rak5005.png
Normal file
Binary file not shown.
After Width: | Height: | Size: 192 KiB |
BIN
static/img/enclosures/3dp-tropho-tbeam.jpg
Normal file
BIN
static/img/enclosures/3dp-tropho-tbeam.jpg
Normal file
Binary file not shown.
After Width: | Height: | Size: 288 KiB |
Loading…
Reference in a new issue