2022-10-27 05:57:49 -07:00
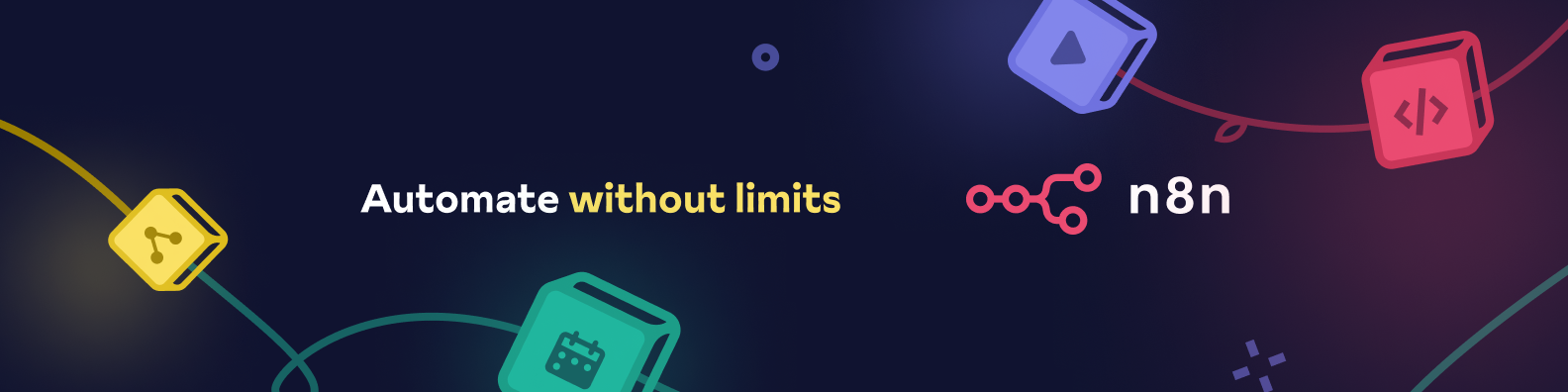
2019-06-23 03:35:23 -07:00
2023-08-16 08:13:57 -07:00
# n8n-node-dev
2019-06-23 03:35:23 -07:00
Currently very simple and not very sophisticated CLI which makes it easier
to create credentials and nodes in TypeScript for n8n.
```
npm install n8n-node-dev -g
```
2019-08-21 14:30:19 -07:00
## Contents
- [Usage ](#usage )
- [Commands ](#commands )
- [Create a node ](#create-a-node )
2022-11-22 05:11:29 -08:00
- [Node Type ](#node-type )
- [Node Type Description ](#node-type-description )
- [Node Properties ](#node-properties )
- [Node Property Options ](#node-property-options )
2019-08-21 14:30:19 -07:00
- [License ](#license )
2019-06-23 03:35:23 -07:00
## Usage
The commandline tool can be started with `n8n-node-dev <COMMAND>`
## Commands
The following commands exist:
### build
Builds credentials and nodes in the current folder and copies them into the
n8n custom extension folder (`~/.n8n/custom/`) unless destination path is
overwritten with `--destination <FOLDER_PATH>`
When "--watch" gets set it starts in watch mode and automatically builds and
copies files whenever they change. To stop press "ctrl + c".
### new
Creates new basic credentials or node of the selected type to have a first starting point.
2019-07-07 10:22:22 -07:00
## Create a node
The easiest way to create a new node is via the "n8n-node-dev" cli. It sets up
all the basics.
A n8n node is a JavaScript file (normally written in TypeScript) which describes
some basic information (like name, description, ...) and also at least one method.
2023-06-26 02:05:05 -07:00
Depending on which method gets implemented defines if it is a regular-, trigger-
2019-07-07 10:22:22 -07:00
or webhook-node.
A simple regular node which:
2022-11-22 05:11:29 -08:00
- defines one node property
2023-06-26 02:05:05 -07:00
- sets its value to all items it receives
2019-07-07 10:22:22 -07:00
would look like this:
File named: `MyNode.node.ts`
2022-11-22 05:11:29 -08:00
2019-07-07 10:22:22 -07:00
```TypeScript
import {
2023-07-12 02:15:38 -07:00
IExecuteFunctions,
2019-07-07 10:22:22 -07:00
INodeExecutionData,
INodeType,
INodeTypeDescription,
} from 'n8n-workflow';
export class MyNode implements INodeType {
description: INodeTypeDescription = {
displayName: 'My Node',
name: 'myNode',
group: ['transform'],
version: 1,
description: 'Adds "myString" on all items to defined value.',
defaults: {
name: 'My Node',
color: '#772244',
},
inputs: ['main'],
outputs: ['main'],
properties: [
// Node properties which the user gets displayed and
// can change on the node.
{
displayName: 'My String',
name: 'myString',
type: 'string',
default: '',
placeholder: 'Placeholder value',
description: 'The description text',
}
]
};
async execute(this: IExecuteFunctions): Promise< INodeExecutionData [ ] [ ] > {
const items = this.getInputData();
let item: INodeExecutionData;
let myString: string;
// Itterates over all input items and add the key "myString" with the
// value the parameter "myString" resolves to.
// (This could be a different value for each item in case it contains an expression)
for (let itemIndex = 0; itemIndex < items.length ; itemIndex + + ) {
myString = this.getNodeParameter('myString', itemIndex, '') as string;
item = items[itemIndex];
item.json['myString'] = myString;
}
2023-09-05 03:59:02 -07:00
return [items];
2019-07-07 10:22:22 -07:00
}
}
```
The "description" property has to be set on all nodes because it contains all
2023-06-26 02:05:05 -07:00
the base information. Additionally all nodes have to have exactly one of the
2020-07-03 06:35:58 -07:00
following methods defined which contains the actual logic:
2019-07-07 10:22:22 -07:00
**Regular node**
2023-06-26 02:05:05 -07:00
Method is called when the workflow gets executed
2022-11-22 05:11:29 -08:00
- `execute` : Executed once no matter how many items
2019-07-07 10:22:22 -07:00
2023-06-26 02:05:05 -07:00
By default, `execute` should always be used, especially when creating a
2023-08-16 08:13:57 -07:00
third-party integration. The reason for this is that it provides much more
2023-06-26 02:05:05 -07:00
flexibility and allows, for example, returning a different number of items than
it received as input. This becomes crucial when a node needs to query data such as _return
all users_. In such cases, the node typically receives only one input item but returns as
many items as there are users. Therefore, when in doubt, it is recommended to use `execute` !
2019-07-07 10:22:22 -07:00
**Trigger node**
2023-06-26 02:05:05 -07:00
Method is called once when the workflow gets activated. It can then trigger workflow runs and provide the necessary data by itself.
2019-07-07 10:22:22 -07:00
2022-11-22 05:11:29 -08:00
- `trigger`
2019-07-07 10:22:22 -07:00
2022-11-22 05:11:29 -08:00
**Webhook node**
2019-07-07 10:22:22 -07:00
2023-06-26 02:05:05 -07:00
Method is called when webhook gets called.
2019-07-07 10:22:22 -07:00
2022-11-22 05:11:29 -08:00
- `webhook`
2019-07-07 10:22:22 -07:00
### Node Type
Property overview
2022-11-22 05:11:29 -08:00
- **description** [required]: Describes the node like its name, properties, hooks, ... see `Node Type Description` bellow.
2023-06-26 02:05:05 -07:00
- **execute** [optional]: Method is called when the workflow gets executed (once).
2022-11-22 05:11:29 -08:00
- **hooks** [optional]: The hook methods.
- **methods** [optional]: Additional methods. Currently only "loadOptions" exists which allows loading options for parameters from external services
2023-06-26 02:05:05 -07:00
- **trigger** [optional]: Method is called once when the workflow gets activated.
- **webhook** [optional]: Method is called when webhook gets called.
2022-11-22 05:11:29 -08:00
- **webhookMethods** [optional]: Methods to setup webhooks on external services.
2019-07-07 10:22:22 -07:00
### Node Type Description
The following properties can be set in the node description:
2022-11-22 05:11:29 -08:00
- **credentials** [optional]: Credentials the node requests access to
- **defaults** [required]: Default "name" and "color" to set on node when it gets created
- **displayName** [required]: Name to display users in Editor UI
- **description** [required]: Description to display users in Editor UI
- **group** [required]: Node group for example "transform" or "trigger"
- **hooks** [optional]: Methods to execute at different points in time like when the workflow gets activated or deactivated
2023-06-26 02:05:05 -07:00
- **icon** [optional]: Icon to display (can be an icon or a font awesome icon)
2022-11-22 05:11:29 -08:00
- **inputs** [required]: Types of inputs the node has (currently only "main" exists) and the amount
- **outputs** [required]: Types of outputs the node has (currently only "main" exists) and the amount
2023-06-26 02:05:05 -07:00
- **outputNames** [optional]: In case a node has multiple outputs, names can be set that users know what data to expect
- **maxNodes** [optional]: If an unlimited number of nodes of that type cannot exist in a workflow, the max-amount can be specified
2022-11-22 05:11:29 -08:00
- **name** [required]: Name of the node (for n8n to use internally, in camelCase)
- **properties** [required]: Properties which get displayed in the Editor UI and can be set by the user
- **subtitle** [optional]: Text which should be displayed underneath the name of the node in the Editor UI (can be an expression)
2023-06-26 02:05:05 -07:00
- **version** [required]: Version of the node. Currently always "1" (integer). For future usage, does not get used yet
2022-11-22 05:11:29 -08:00
- **webhooks** [optional]: Webhooks the node should listen to
2019-07-07 10:22:22 -07:00
### Node Properties
The following properties can be set in the node properties:
2022-11-22 05:11:29 -08:00
- **default** [required]: Default value of the property
- **description** [required]: Description that is displayed to users in the Editor UI
- **displayName** [required]: Name that is displayed to users in the Editor UI
- **displayOptions** [optional]: Defines logic to decide if a property should be displayed or not
- **name** [required]: Name of the property (for n8n to use internally, in camelCase)
- **options** [optional]: The options the user can select when type of property is "collection", "fixedCollection" or "options"
- **placeholder** [optional]: Placeholder text that is displayed to users in the Editor UI
- **type** [required]: Type of the property. If it is for example a "string", "number", ...
- **typeOptions** [optional]: Additional options for type. Like for example the min or max value of a number
- **required** [optional]: Defines if the value has to be set or if it can stay empty
2019-07-07 10:22:22 -07:00
### Node Property Options
2023-06-26 02:05:05 -07:00
The following properties can be set in the node property options:
2019-07-07 10:22:22 -07:00
2020-07-03 06:35:58 -07:00
All properties are optional. However, most only work when the node-property is of a specfic type.
2019-07-07 10:22:22 -07:00
2023-06-26 02:05:05 -07:00
- **alwaysOpenEditWindow** [type: json]: If set then the "Editor Window" will always open when the user tries to edit the field. Helpful if long text is typically used in the property
2022-08-03 04:34:49 -07:00
- **loadOptionsMethod** [type: options]: Method to use to load options from an external service
- **maxValue** [type: number]: Maximum value of the number
- **minValue** [type: number]: Minimum value of the number
- **multipleValues** [type: all]: If set the property gets turned into an Array and the user can add multiple values
2023-06-26 02:05:05 -07:00
- **multipleValueButtonText** [type: all]: Custom text for add button in case "multipleValues" were set
- **numberPrecision** [type: number]: The precision of the number. By default, it is "0" and will only allow integers
- **password** [type: string]: If a password field should be displayed (normally only used by credentials because all node data is not encrypted and gets saved in clear-text)
2022-08-03 04:34:49 -07:00
- **rows** [type: string]: Number of rows the input field should have. By default it is "1"
2019-07-07 10:22:22 -07:00
2019-06-23 03:35:23 -07:00
## License
2022-03-17 02:15:24 -07:00
n8n is [fair-code ](http://faircode.io ) distributed under the [**Sustainable Use License** ](https://github.com/n8n-io/n8n/blob/master/packages/cli/LICENSE.md ).
2019-10-04 11:40:23 -07:00
2023-04-20 09:49:09 -07:00
Proprietary licenses are available for enterprise customers. [Get in touch ](mailto:license@n8n.io )
2022-08-03 04:34:49 -07:00
Additional information about the license can be found in the [docs ](https://docs.n8n.io/reference/license/ ).